python对中文txt进行词云分析生成的图片没有字而是一个个方框请问这是怎么回事?import jieba import wordcloud with open('a.txt','r',encoding="utf-8") as f: temp = f.read() words = jieba.lcut(temp) words = " ".join(words) pl = wordcloud.WordCloud( width = 1000,height = 1000, background_color = "white", #font_path = r"C:\\Windows\\Fonts\\TEMPSITC.TTF" ) pl.generate(temp) pl.to_file("C:\\Users\\86150\\Desktop\\1.png")并检查一下代码的错误
时间: 2023-07-07 14:40:39 浏览: 152
这可能是因为你的字体库没有支持中文字符。你可以尝试将`font_path`参数设置为一个支持中文的字体文件路径。例如:
```
font_path = r"C:\Windows\Fonts\SimSun.ttf"
```
另外,你需要将`pl.generate(temp)`中的`temp`改为`words`,以使用中文分词后的单词列表生成词云。修改后的代码如下:
```
import jieba
import wordcloud
with open('a.txt','r',encoding="utf-8") as f:
temp = f.read()
words = jieba.lcut(temp)
words = " ".join(words)
pl = wordcloud.WordCloud(
width = 1000,height = 1000,
background_color = "white",
font_path = r"C:\Windows\Fonts\SimSun.ttf"
)
pl.generate(words)
pl.to_file("C:\\Users\\86150\\Desktop\\1.png")
```
注意,你需要将`font_path`参数中的路径改为你自己的中文字体文件路径。
相关问题
python利用jieba和wordcloud生成词云图
1. 安装jieba和wordcloud库
```python
!pip install jieba
!pip install wordcloud
```
2. 导入库
```python
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
```
3. 导入文本数据
```python
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
```
4. 使用jieba进行分词
```python
text = ' '.join(jieba.cut(text))
```
5. 生成词云图
```python
wordcloud = WordCloud(font_path='msyh.ttc',
background_color='white',
width=800,
height=600).generate(text)
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
完整代码:
```python
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
text = ' '.join(jieba.cut(text))
wordcloud = WordCloud(font_path='msyh.ttc',
background_color='white',
width=800,
height=600).generate(text)
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
python利用jieba和wordcloud生成词云图怎么做
Python利用jieba和wordcloud生成词云图的方法如下:
1. 安装jieba和wordcloud库:
```
pip install jieba
pip install wordcloud
```
2. 导入需要的库:
```python
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
```
3. 读取文本文件并进行分词:
```python
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
words = jieba.cut(text)
```
4. 将分好的词转换为字符串并使用WordCloud生成词云图:
```python
wordcloud = WordCloud(font_path='msyh.ttc', width=800, height=600, background_color='white').generate(' '.join(words))
plt.imshow(wordcloud)
plt.axis('off')
plt.show()
```
其中,`font_path`指定字体文件路径,`width`和`height`为词云图的宽度和高度,`background_color`为背景颜色,默认为黑色。最后一步使用`plt.imshow()`显示词云图,并使用`plt.axis('off')`去掉坐标轴,最后使用`plt.show()`显示词云图。
完整代码示例:
```python
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文本文件并进行分词
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
words = jieba.cut(text)
# 生成词云图
wordcloud = WordCloud(font_path='msyh.ttc', width=800, height=600, background_color='white').generate(' '.join(words))
# 显示词云图
plt.imshow(wordcloud)
plt.axis('off')
plt.show()
```
阅读全文
相关推荐
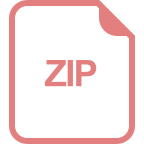
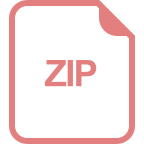
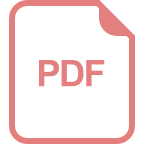
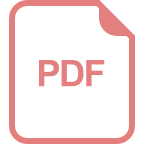
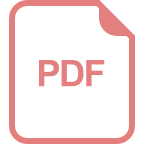
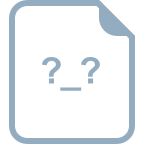
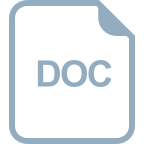







