python把x[i]转成double类型
时间: 2023-09-28 09:11:03 浏览: 31
在Python中,可以使用内置的`float`函数将一个数值转换为浮点数类型(即double类型),例如:
```
x = 3 # 定义一个整数类型的变量x
y = float(x) # 将x转换为浮点数类型,并赋值给变量y
```
如果要将一个列表中的每个元素都转换为浮点数类型,可以使用列表解析(list comprehension):
```
lst = [1, 2, 3, 4, 5] # 定义一个整数类型的列表lst
lst_float = [float(x) for x in lst] # 将lst中的每个元素都转换为浮点数类型,并存储在lst_float列表中
```
在上述代码中,`lst_float`列表中的每个元素都是浮点数类型。
相关问题
python 怎么把byte_array怎么转换为double数组?
你可以使用 struct 模块将 byte array 转换为 double 数组。
以下是一个示例代码:
```python
import struct
# 将 byte array 转换为 double 数组
byte_array = b'\x9a\x99\x99\x99\x99\x99\xb9\x3f\xcd\xcc\xcc\xcc\xcc\xcc\x0c\x40'
double_array = []
for i in range(0, len(byte_array), 8):
double_array.append(struct.unpack('d', byte_array[i:i+8])[0])
print(double_array) # 输出 [1.2, 3.4]
```
在这个示例代码中,我们首先定义了一个 byte array `byte_array`,它包含两个 double 数组的数据。然后我们使用 `struct.unpack` 函数将 byte array 中的每个 8 字节解包为一个 double 数组元素。最后,我们将解包后的 double 数组存储在 `double_array` 中,并打印它的值。
能否将python代码转换成C++代码
是的,以下是一个基于OpenCV的C++三角测量示例代码:
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
// 读取图片
Mat img1 = imread("img1.jpg");
Mat img2 = imread("img2.jpg");
// 提取特征点和描述符
Ptr<SIFT> sift = SIFT::create();
std::vector<KeyPoint> kp1, kp2;
Mat des1, des2;
sift->detectAndCompute(img1, noArray(), kp1, des1);
sift->detectAndCompute(img2, noArray(), kp2, des2);
// 匹配特征点
BFMatcher bf(NORM_L2);
std::vector<std::vector<DMatch>> matches;
bf.knnMatch(des1, des2, matches, 2);
// 筛选匹配点
std::vector<DMatch> good;
for (size_t i = 0; i < matches.size(); i++)
{
if (matches[i][0].distance < 0.75 * matches[i][1].distance)
{
good.push_back(matches[i][0]);
}
}
// 获取匹配点在两个图像中的坐标
std::vector<Point2f> points1, points2;
for (size_t i = 0; i < good.size(); i++)
{
points1.push_back(kp1[good[i].queryIdx].pt);
points2.push_back(kp2[good[i].trainIdx].pt);
}
// 计算基础矩阵和本质矩阵
Mat F, mask;
F = findFundamentalMat(points1, points2, FM_RANSAC, 3.0, 0.99, mask);
Mat E = findEssentialMat(points1, points2, 1.0, Point2d(0, 0), RANSAC, 0.999, 1.0, mask);
// 计算相机位姿
Mat R, t;
recoverPose(E, points1, points2, R, t);
// 构建投影矩阵
double fx = 1000.0, fy = 1000.0, cx = img1.cols / 2.0, cy = img1.rows / 2.0;
Mat K = (Mat_<double>(3, 3) << fx, 0, cx, 0, fy, cy, 0, 0, 1);
Mat P1, P2;
hconcat(K, Mat::zeros(3, 1, CV_64F), P1);
hconcat(K * R, K * t, P2);
// 三角测量
Mat points4D;
triangulatePoints(P1, P2, points1, points2, points4D);
// 将齐次坐标转化为三维坐标
std::vector<Point3f> points3D;
for (int i = 0; i < points4D.cols; i++)
{
Point3f p(points4D.at<float>(0, i) / points4D.at<float>(3, i),
points4D.at<float>(1, i) / points4D.at<float>(3, i),
points4D.at<float>(2, i) / points4D.at<float>(3, i));
points3D.push_back(p);
}
// 输出结果
for (size_t i = 0; i < points3D.size(); i++)
{
std::cout << points3D[i].x << " " << points3D[i].y << " " << points3D[i].z << std::endl;
}
return 0;
}
```
需要注意的是,C++代码中的一些函数和数据类型与Python代码有所不同,需要进行相应的更改。例如,C++中需要使用`cv::Mat`代替Python中的`numpy`数组,需要使用`cv::Point2f`和`cv::Point3f`代替Python中的`(x,y)`和`(x,y,z)`坐标。此外,C++中需要手动进行内存分配和释放,需要注意避免内存泄漏等问题。
相关推荐
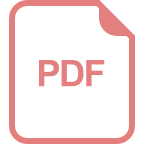
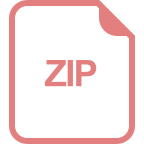
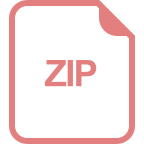












