爬取给定网址中的文本信息,并存储到doc格式文件中,增加头文件反爬,写成函数python代码
时间: 2023-08-31 17:31:05 浏览: 170
### 回答1:
好的,我来帮你写一个函数。首先需要使用 Python 的 `requests` 库来发送 HTTP 请求并获取网页内容,再使用 `BeautifulSoup` 库来解析网页内容。代码如下:
```
import requests
from bs4 import BeautifulSoup
def crawl_text(url, headers):
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
text = soup.get_text()
return text
```
然后,你可以使用 Python 的 `docx` 库来将文本存储到 doc 格式文件中。代码如下:
```
import docx
def save_to_doc(text, filename):
document = docx.Document()
document.add_paragraph(text)
document.save(filename)
```
最后,你可以将这两个函数合并到一起,并增加头文件来实现反爬。代码如下:
```
import requests
from bs4 import BeautifulSoup
import docx
def crawl_and_save(url, headers, filename):
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
text = soup.get_text()
document = docx.Document()
document.add_paragraph(text)
document.save(filename)
```
希望这些代码能帮到你!
### 回答2:
在Python中实现爬取给定网址中的文本信息并存储为doc格式文件,我会提供一个示例函数代码如下:
```python
import requests
from bs4 import BeautifulSoup
import docx
def crawl_and_save(url, filename):
# 设置头文件,模拟浏览器请求
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.82 Safari/537.36"
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
# 解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 提取文本信息
text = soup.get_text()
# 创建一个docx文档对象
doc = docx.Document()
# 将提取到的文本信息写入文档
doc.add_paragraph(text)
# 保存文档
doc.save(filename)
print(f"文本已保存到{filename}文件中!")
else:
print("请求失败!")
# 测试示例
crawl_and_save("http://www.example.com", "example.docx")
```
以上函数通过使用requests库发送带有自定义头文件(User-Agent)的HTTP请求模拟浏览器的行为,以增加反爬虫的效果。然后使用BeautifulSoup库解析网页内容,提取文本信息。最后,使用docx库创建一个docx文档对象,并将提取到的文本信息写入文档,最终保存为doc格式的文件。在函数的参数中传入需要爬取的网址和保存文档的文件名即可运行。
### 回答3:
要实现爬取给定网址中的文本信息并存储到doc格式文件中,可以使用python中的requests库进行网页请求,使用beautifulsoup库进行网页解析,并借助python-docx库实现doc文件的创建和写入。
下面是一个函数的示例代码:
```python
import requests
from bs4 import BeautifulSoup
from docx import Document
def crawl_and_save(url, headers, file_path):
# 发送带有头文件的请求
response = requests.get(url, headers=headers)
# 使用beautifulsoup解析网页内容
soup = BeautifulSoup(response.content, 'html.parser')
# 创建doc文件
doc = Document()
# 找到所有的文本信息
texts = soup.find_all(text=True)
# 将文本信息写入doc文件
for text in texts:
doc.add_paragraph(text)
# 保存doc文件
doc.save(file_path)
# 示例调用
url = 'https://example.com'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'}
file_path = 'output.docx'
crawl_and_save(url, headers, file_path)
```
在上述代码中,我们通过requests库发送带有头文件的请求,以便模拟真实浏览器的行为,避免被网站反爬机制拦截。然后使用beautifulsoup库解析网页内容,将文本信息提取出来,并逐个写入doc文件中。最后保存doc文件到指定位置。
请注意,需要安装requests、beautifulsoup和python-docx库才能运行以上代码。你可以使用`pip install requests beautifulsoup4 python-docx`来安装这些库。
相关推荐
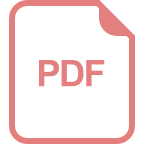
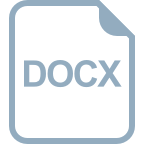














