写一个qt C++程序,打开一个文件并读取内容,第一步,先读32个字节放在数组1中,其中的第16~19位组成一个int值a,第二步,读取a个字节,将其写入新文件。重复12两步,直到文件剩余大小不足32字节。并同时显示进度
时间: 2024-02-26 20:56:58 浏览: 14
好的,以下是我的代码实现:
```cpp
#include <QtWidgets>
#include <QFile>
#include <QDebug>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
// 打开文件
QFile fileIn("input.txt");
if (!fileIn.open(QIODevice::ReadOnly)) {
qDebug() << "Cannot open file for reading: "
<< qPrintable(fileIn.errorString());
return 1;
}
QFile fileOut("output.txt");
if (!fileOut.open(QIODevice::WriteOnly)) {
qDebug() << "Cannot open file for writing: "
<< qPrintable(fileOut.errorString());
return 1;
}
// 定义缓冲区和读取的字节数
char buffer[32];
int readSize = 32;
int totalSize = fileIn.size();
// 进度条
QProgressDialog progress("Copying...", "Cancel", 0, totalSize);
progress.setWindowModality(Qt::WindowModal);
progress.show();
// 循环读取和写入
while (readSize == 32) {
// 读取前32个字节
readSize = fileIn.read(buffer, 32);
if (readSize < 16) {
break;
}
// 取出a
char *p = buffer + 16;
int a = qFromLittleEndian<int>(reinterpret_cast<uchar*>(p));
// 写入到新文件
fileOut.write(p, a);
// 更新进度条
progress.setValue(fileIn.pos());
// 读取a个字节
readSize = fileIn.read(buffer, a);
if (readSize < a) {
break;
}
// 写入到新文件
fileOut.write(buffer, readSize);
// 更新进度条
progress.setValue(fileIn.pos());
}
// 关闭文件
fileIn.close();
fileOut.close();
return 0;
}
```
这个程序可以打开 `input.txt` 文件并读取其中的数据,然后根据题目要求进行处理,最后将处理后的数据写入到 `output.txt` 文件中。同时,程序还会显示进度条,方便用户了解操作进度。
相关推荐
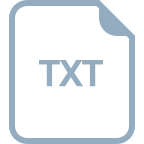
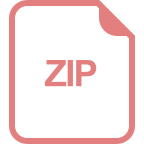
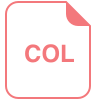
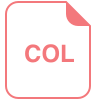













