python写一个好友管理系统,具有好友管理系统的基本功能,包括添加好友、删除好友、备注好友、展示好友等。
时间: 2024-10-23 12:07:04 浏览: 14
在Python中创建一个简单的好友管理系统可以使用字典作为数据结构,因为字典非常适合存储键值对,其中键可以代表用户ID,值可以包含更多的信息如用户名、备注等。下面是一个基础的实现:
```python
class FriendManager:
def __init__(self):
self.friends = {} # 使用字典存储好友信息
def add_friend(self, user_id, username, note=""):
"""
添加好友
:param user_id: 用户ID
:param username: 好友名字
:param note: 备注(可选)
"""
if user_id not in self.friends:
self.friends[user_id] = {'username': username, 'note': note}
print(f"成功添加好友 {username},ID: {user_id}")
else:
print("该用户ID已存在")
def remove_friend(self, user_id):
"""
删除好友
:param user_id: 用户ID
"""
if user_id in self.friends:
del self.friends[user_id]
print(f"成功移除好友 {self.friends.get(user_id, {}).get('username', '未知')}, ID: {user_id}")
else:
print("未找到该好友")
def update_friend_note(self, user_id, new_note):
"""
更新好友备注
:param user_id: 用户ID
:param new_note: 新备注
"""
if user_id in self.friends:
self.friends[user_id]['note'] = new_note
print(f"成功更新好友 {user_id} 的备注为: {new_note}")
else:
print("未找到该好友")
def show_friends(self):
"""
展示所有好友
"""
for friend_id, details in self.friends.items():
print(f"好友ID: {friend_id}, 名称: {details['username']}, 备注: {details.get('note', '无备注')}")
# 使用示例
fm = FriendManager()
fm.add_friend(1, "小明", "技术宅")
fm.add_friend(2, "小红")
fm.update_friend_note(1, "编程爱好者")
fm.show_friends()
fm.remove_friend(2)
```
阅读全文
相关推荐
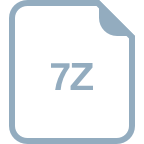
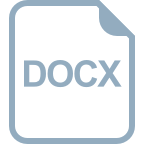
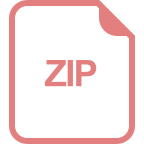














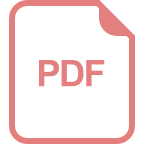