用决策树对minist数据集进行识别python代码
时间: 2024-02-22 09:55:16 浏览: 22
以下是使用决策树对MNIST数据集进行识别的Python代码:
```python
from sklearn.datasets import fetch_openml
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
from sklearn.metrics import accuracy_score
# 获取MNIST数据集
mnist = fetch_openml('mnist_784')
X, y = mnist["data"], mnist["target"]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 训练决策树模型
tree_clf = DecisionTreeClassifier(random_state=42)
tree_clf.fit(X_train, y_train)
# 在测试集上评估模型
y_pred = tree_clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
```
注意,这段代码使用的是sklearn库内置的MNIST数据集。如果你使用的是其他来源的MNIST数据集,可能需要对数据进行一些预处理。
相关问题
svm识别minist数据集python代码
以下是使用 SVM 对 MNIST 数据集进行分类的 Python 代码示例:
```python
import numpy as np
from sklearn import datasets, svm
from sklearn.model_selection import train_test_split
# 加载 MNIST 数据集
digits = datasets.load_digits()
# 分割数据集为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(digits.data, digits.target, test_size=0.3, random_state=42)
# 创建 SVM 分类器
clf = svm.SVC(kernel='linear', C=1, gamma='scale')
# 训练 SVM 分类器
clf.fit(X_train, y_train)
# 在测试集上进行预测
y_pred = clf.predict(X_test)
# 计算模型准确率
accuracy = np.mean(y_pred == y_test)
print("Accuracy:", accuracy)
```
在这段代码中,我们首先导入必要的库,然后加载 MNIST 数据集并将其分割为训练集和测试集。接下来,我们创建一个 SVM 分类器,并使用训练集训练它。然后,我们在测试集上进行预测,并计算模型的准确率。最后,我们将准确率打印出来。
用感知器进行minist数据集手写数字识别matlab代码
以下是使用感知器进行手写数字识别的 MATLAB 代码:
1. 导入数据集
首先,需要导入 MINIST 数据集。这个数据集包含了很多手写数字的图像和对应的标签。可以通过以下代码导入:
```matlab
load('mnist.mat'); % 导入 MINIST 数据集
```
2. 数据预处理
由于感知器只能接受一维向量作为输入,因此需要将每个手写数字图像转换为一维向量。同时,还需要将图像的像素值从 0-255 转换为 -1 到 1 之间的实数。可以通过以下代码实现:
```matlab
% 将图像转换为一维向量
train_data = reshape(train_images, [], 784);
test_data = reshape(test_images, [], 784);
% 将像素值归一化到 -1 到 1 之间
train_data = double(train_data) / 127.5 - 1;
test_data = double(test_data) / 127.5 - 1;
```
另外,需要将标签转换为独热编码,以便在训练过程中使用。可以通过以下代码实现:
```matlab
train_labels = zeros(60000, 10);
test_labels = zeros(10000, 10);
for i = 1:60000
train_labels(i, train_labels_raw(i) + 1) = 1;
end
for i = 1:10000
test_labels(i, test_labels_raw(i) + 1) = 1;
end
```
3. 定义感知器模型
感知器模型包括输入层、隐藏层和输出层。在这个例子中,输入层有 784 个神经元,隐藏层有 32 个神经元,输出层有 10 个神经元(分别对应 0-9 十个数字)。可以通过以下代码定义模型:
```matlab
input_size = 784; % 输入层大小
hidden_size = 32; % 隐藏层大小
output_size = 10; % 输出层大小
% 初始化权重和偏置
W1 = randn(input_size, hidden_size) / sqrt(input_size);
b1 = zeros(1, hidden_size);
W2 = randn(hidden_size, output_size) / sqrt(hidden_size);
b2 = zeros(1, output_size);
% 定义前向传播函数
forward = @(x) softmax(x * W2 + b2);
```
其中,softmax 函数用于将输出层的输出转换为概率分布。
4. 训练模型
可以使用随机梯度下降算法训练感知器模型。每次迭代时,从训练集中随机选择一个样本,计算前向传播和反向传播,然后更新权重和偏置。可以通过以下代码实现:
```matlab
learning_rate = 0.1; % 学习率
batch_size = 32; % 批量大小
num_epochs = 10; % 迭代次数
num_batches = ceil(size(train_data, 1) / batch_size);
for epoch = 1:num_epochs
for batch = 1:num_batches
% 选择一个随机批量
idx = randperm(size(train_data, 1), batch_size);
x = train_data(idx, :);
y = train_labels(idx, :);
% 前向传播
hidden = tanh(x * W1 + b1);
output = forward(hidden);
% 反向传播
error = output - y;
dW2 = hidden' * error;
db2 = sum(error, 1);
dhidden = (1 - hidden.^2) .* (error * W2');
dW1 = x' * dhidden;
db1 = sum(dhidden, 1);
% 更新权重和偏置
W2 = W2 - learning_rate * dW2;
b2 = b2 - learning_rate * db2;
W1 = W1 - learning_rate * dW1;
b1 = b1 - learning_rate * db1;
end
% 在测试集上评估模型表现
hidden = tanh(test_data * W1 + b1);
output = forward(hidden);
[~, pred] = max(output, [], 2);
accuracy = mean(pred == test_labels_raw);
fprintf('Epoch %d, Accuracy: %f\n', epoch, accuracy);
end
```
5. 使用模型进行预测
训练完成后,可以使用模型进行预测。可以通过以下代码实现:
```matlab
hidden = tanh(test_data * W1 + b1);
output = forward(hidden);
[~, pred] = max(output, [], 2);
```
其中,`pred` 变量包含了模型在测试集上的预测结果。可以将其与真实标签进行比较,计算模型的准确率。
相关推荐
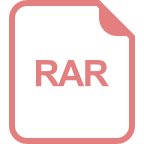
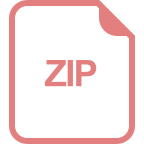












