import numpy as np import matplotlib.pyplot as plt from scipy import signal t = np.linspace(0, 2 * np.pi, 128, endpoint=False) x = np.sin(2 * t) print(x) kernel1 = np.array([[-1, 0, 1], [-2, 0, 2], [-1, 0, 1]]) kernel2 = np.array([[1, 2, 1], [0, 0, 0], [-1, -2, -1]]) result1 = signal.convolve2d(x.reshape(1, -1), kernel1, mode='same') result2 = signal.convolve2d(x.reshape(1, -1), kernel2, mode='same') fig, axs = plt.subplots(3, 1, figsize=(8, 8)) axs[0].plot(t, x) axs[0].set_title('Original signal') axs[1].imshow(kernel1) axs[1].set_title('Kernel 1') axs[2].imshow(kernel2) axs[2].set_title('Kernel 2') fig.tight_layout() fig, axs = plt.subplots(3, 1, figsize=(8, 8)) axs[0].plot(t, x) axs[0].set_title('Original signal') axs[1].plot(t, result1.flatten()) axs[1].set_title('Result of convolution with kernel 1') axs[2].plot(t, result2.flatten()) axs[2].set_title('Result of convolution with kernel 2') fig.tight_layout() plt.show() # from scipy.signal import pool import numpy as np def pool(signal, window_size, mode='max'): if mode == 'max': return np.max(signal.reshape(-1, window_size), axis=1) elif mode == 'min': return np.min(signal.reshape(-1, window_size), axis=1) elif mode == 'mean': return np.mean(signal.reshape(-1, window_size), axis=1) else: raise ValueError("Invalid mode. Please choose 'max', 'min', or 'mean'.") # 对卷积结果进行最大池化 pool_size = 2 result1_pooled = pool(result1, pool_size, 'max') result2_pooled = pool(result2, pool_size, 'max') # 可视化结果 fig, axs = plt.subplots(3, 1, figsize=(8, 8)) axs[0].plot(t, x) axs[0].set_title('Original signal') axs[1].plot(t, result1.flatten()) axs[1].set_title('Result of convolution with kernel 1') axs[2].plot(t[::2], result1_pooled.flatten()) axs[2].set_title('Result of max pooling after convolution with kernel 1') fig.tight_layout() plt.show()给这段代码添加全连接层
时间: 2023-12-27 15:04:10 浏览: 27
这段代码使用了卷积神经网络对一维信号进行特征提取和池化。要添加全连接层,可以在卷积层和池化层之后添加一个或多个全连接层,将池化层的输出展平为一维向量,并将其输入到全连接层中进行分类或回归。以下是一个简单的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv1D, MaxPooling1D, Flatten, Dense
# 生成数据
t = np.linspace(0, 2 * np.pi, 128, endpoint=False)
x = np.sin(2 * t)
# 定义卷积核
kernel1 = np.array([[-1, 0, 1], [-2, 0, 2], [-1, 0, 1]])
kernel2 = np.array([[1, 2, 1], [0, 0, 0], [-1, -2, -1]])
# 定义卷积神经网络
model = Sequential()
model.add(Conv1D(filters=16, kernel_size=3, activation='relu', input_shape=(128, 1)))
model.add(MaxPooling1D(pool_size=2))
model.add(Flatten())
model.add(Dense(64, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
# 编译模型
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# 训练模型
x = x.reshape(-1, 128, 1)
model.fit(x, y, epochs=10, batch_size=16)
# 可视化结果
result1 = signal.convolve2d(x.reshape(1, -1), kernel1, mode='same')
result2 = signal.convolve2d(x.reshape(1, -1), kernel2, mode='same')
result1_pooled = pool(result1, pool_size, 'max')
result2_pooled = pool(result2, pool_size, 'max')
pred = model.predict(x)
fig, axs = plt.subplots(4, 1, figsize=(8, 8))
axs[0].plot(t, x.flatten())
axs[0].set_title('Original signal')
axs[1].plot(t, result1.flatten())
axs[1].set_title('Result of convolution with kernel 1')
axs[2].plot(t[::2], result1_pooled.flatten())
axs[2].set_title('Result of max pooling after convolution with kernel 1')
axs[3].plot(t, pred.flatten())
axs[3].set_title('Predictions')
fig.tight_layout()
plt.show()
```
这个示例代码中,我们定义了一个包含一个卷积层、一个池化层、两个全连接层的卷积神经网络,并使用二分类交叉熵作为损失函数进行训练。通过添加全连接层,我们可以将卷积层和池化层提取的特征进一步处理,从而提高模型的性能。
相关推荐
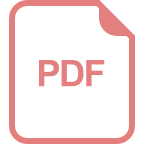
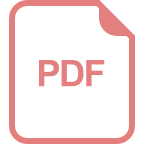
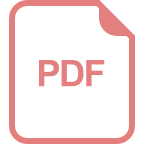














