如何利用js将一张图片均分成四等份,并放到数组里
时间: 2023-08-18 12:08:58 浏览: 153
要将一张图片均分成四等份,并将每个部分存储在数组中,你可以使用 JavaScript 和 HTML5 的 `<canvas>` 元素来实现。下面是一个示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>将图片均分成四等份并存储在数组中</title>
<style>
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<img id="sourceImage" src="path/to/your/image.jpg" style="display: none;">
<script>
window.onload = function() {
var sourceImage = document.getElementById('sourceImage');
var canvas1 = document.createElement('canvas');
var canvas2 = document.createElement('canvas');
var canvas3 = document.createElement('canvas');
var canvas4 = document.createElement('canvas');
var ctx1 = canvas1.getContext('2d');
var ctx2 = canvas2.getContext('2d');
var ctx3 = canvas3.getContext('2d');
var ctx4 = canvas4.getContext('2d');
// 将图片绘制到 canvas1
ctx1.drawImage(sourceImage, 0, 0, sourceImage.width / 2, sourceImage.height / 2, 0, 0, canvas1.width, canvas1.height);
// 将图片绘制到 canvas2
ctx2.drawImage(sourceImage, sourceImage.width / 2, 0, sourceImage.width / 2, sourceImage.height / 2, 0, 0, canvas2.width, canvas2.height);
// 将图片绘制到 canvas3
ctx3.drawImage(sourceImage, 0, sourceImage.height / 2, sourceImage.width / 2, sourceImage.height / 2, 0, 0, canvas3.width, canvas3.height);
// 将图片绘制到 canvas4
ctx4.drawImage(sourceImage, sourceImage.width / 2, sourceImage.height / 2, sourceImage.width / 2, sourceImage.height / 2, 0, 0, canvas4.width, canvas4.height);
var imageParts = [
canvas1.toDataURL(), // 将 canvas1 转换为图片数据URL
canvas2.toDataURL(), // 将 canvas2 转换为图片数据URL
canvas3.toDataURL(), // 将 canvas3 转换为图片数据URL
canvas4.toDataURL() // 将 canvas4 转换为图片数据URL
];
console.log(imageParts); // 输出存储了四等份图片的数组
};
</script>
</body>
</html>
```
在这个示例中,你需要将 `path/to/your/image.jpg` 替换为你自己的图片路径。这段代码将会创建四个等大小的 `<canvas>` 元素,并将原始图片分成四等份分别绘制在不同的 `<canvas>` 中。然后,使用 `toDataURL()` 方法将每个 `<canvas>` 转换为图片数据URL,并存储在 `imageParts` 数组中。
请注意,由于 `toDataURL()` 方法返回的是图片的 base64 编码字符串,所以存储在数组中的数据也都是 base64 编码的字符串。如果需要使用这些图片数据进行进一步的处理或显示,你可能需要进行相应的解码操作。
阅读全文
相关推荐
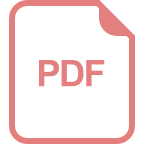










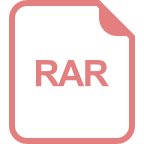
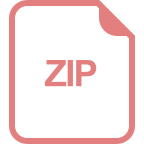