selenium怎么识别图片验证码
时间: 2023-08-30 11:07:23 浏览: 143
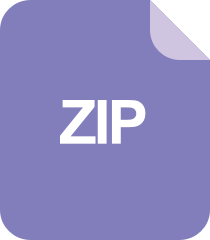
图片验证码识别
Selenium可以通过以下步骤来识别图片验证码:
1. 首先,使用Selenium定位到包含验证码图片的元素。
2. 使用Selenium的`get_attribute`方法获取验证码图片的src属性值。
3. 使用Python的`requests`库发送HTTP请求获取验证码图片的内容。
4. 使用第三方库(如Pillow或OpenCV)加载验证码图片,并进行预处理(如灰度化、二值化等)。
5. 使用第三方库(如Tesseract或pytesseract)对预处理后的图片进行文本识别,获取验证码的文本。
6. 将识别到的验证码文本输入到相应的输入框中,完成验证码的自动识别。
需要注意的是,验证码的复杂程度和图片质量可能会影响识别的准确性。在某些情况下,可能需要使用其他方法(如机器学习模型)来提高识别的成功率。
阅读全文
相关推荐



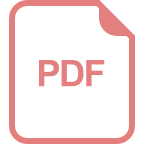
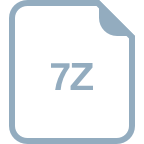
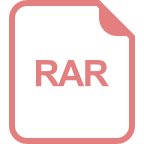
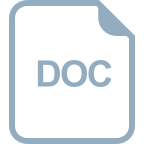
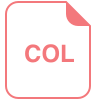
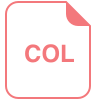






