strptime() argument 1 must be str, not Timestamp
时间: 2024-09-22 16:01:34 浏览: 64
`strptime()` 是 Python 的内置函数,用于将字符串解析为日期和时间,它的第一个参数通常应该是包含日期时间信息的字符串。错误消息 "argument 1 must be str, not Timestamp" 意味着在调用 `strptime()` 函数时,你传递给它的是一个 `Timestamp` 对象而不是字符串。
例如,如果你有类似这样的代码:
```python
from datetime import datetime, timezone
timestamp = datetime.now(timezone.utc)
formatted_date = datetime.strptime(timestamp, "%Y-%m-%d %H:%M:%S")
```
在这个例子中,`timestamp` 是一个 `datetime` 对象,不是字符串,所以你需要先将其转换为字符串再进行格式化。正确的做法应该是:
```python
timestamp_str = timestamp.strftime("%Y-%m-%d %H:%M:%S") # 将Timestamp转换为字符串
formatted_date = datetime.strptime(timestamp_str, "%Y-%m-%d %H:%M:%S")
```
相关问题
报错strptime() argument 1 must be str, not Timestamp
这个错误提示表明在使用strptime()函数时,第一个参数需要传入字符串类型,而不是Pandas中的Timestamp类型。
解决方法:
1.将Timestamp类型转换为字符串类型:
```python
import pandas as pd
from datetime import datetime
df = pd.DataFrame({'date': pd.date_range('2022-01-01', periods=3)})
df['date_str'] = df['date'].apply(lambda x: x.strftime('%Y-%m-%d %H:%M:%S'))
df['date_parsed'] = pd.to_datetime(df['date_str'], format='%Y-%m-%d %H:%M:%S')
print(df)
```
输出:
```
date date_str date_parsed
0 2022-01-01 2022-01-01 00:00:00 2022-01-01 00:00:00
1 2022-01-02 2022-01-02 00:00:00 2022-01-02 00:00:00
2 2022-01-03 2022-01-03 00:00:00 2022-01-03 00:00:00
```
2.使用Pandas的to_datetime()函数直接将Timestamp类型转换为DateTime类型:
```python
import pandas as pd
df = pd.DataFrame({'date': pd.date_range('2022-01-01', periods=3)})
df['date_parsed'] = pd.to_datetime(df['date'], format='%Y-%m-%d %H:%M:%S')
print(df)
```
输出:
```
date date_parsed
0 2022-01-01 2022-01-01 00:00:00
1 2022-01-02 2022-01-02 00:00:00
2 2022-01-03 2022-01-03 00:00:00
```
TypeError: strptime() argument 1 must be str, not Timestamp
这个错误通常发生在使用Python的datetime库中,当你将一个pandas中的Timestamp对象作为参数传递给datetime库中的strptime()函数时,就会出现这个错误。
解决方法是将Timestamp对象转换为字符串类型,可以使用strftime()函数将其转换为指定格式的字符串,然后再将其传递给strptime()函数。例如:
```python
import datetime
import pandas as pd
# 创建一个Timestamp对象
ts = pd.Timestamp('2021-01-01 12:00:00')
# 将Timestamp对象转换为字符串类型
ts_str = ts.strftime('%Y-%m-%d %H:%M:%S')
# 使用datetime库中的strptime()函数进行转换
dt = datetime.datetime.strptime(ts_str, '%Y-%m-%d %H:%M:%S')
```
这样就可以避免出现TypeError: strptime() argument 1 must be str, not Timestamp这个错误了。
阅读全文
相关推荐
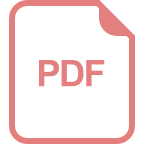
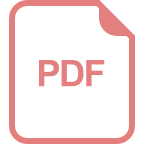











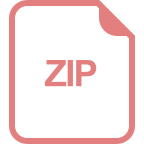
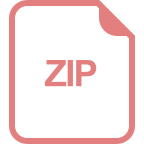