用vhdl设计4位二进制加减可逆计数器,要求具有异步清零功能,清零信号高电平有效
时间: 2024-10-27 13:05:20 浏览: 70
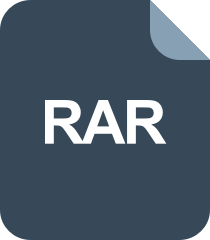
基于VHDL和QuartusII的十进制可逆计数器

在 VHDL 设计中,创建一个四位二进制加减可逆计数器,包括异步清零功能,可以分为以下几个步骤:
1. **模块声明**:
首先,你需要定义一个模块,比如 `FourBitReversibleCounter`,包含输入端口如 `clk` (时钟)、`reset` (异步清零信号,当高电平时清零) 和输出端口 `count_out` (四位二进制计数值)。
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity FourBitReversibleCounter is
Port(
clk : in STD_LOGIC;
reset : in STD_LOGIC;
count_out : out STD_LOGIC_VECTOR(3 downto 0)
);
end FourBitReversibleCounter;
```
2. **结构体定义**:
定义内部状态机的行为,通常会使用进程 (process) 来处理计数和控制逻辑。这里需要两个状态:正常计数和清零。
```vhdl
architecture Behavioral of FourBitReversibleCounter is
begin
process(clk, reset)
begin
if rising_edge(clk) then
-- 当计数器不是最高位清零时,根据当前状态执行加法或减法操作
if reset = '1' or count_out(3) = '0' then
count_out <= "0000"; -- 异步清零,所有位置零
else
-- 加法计数(从0到15)
if count_out(3) = '0' then
count_out <= count_out + X"0001";
-- 减法计数(从14到0)
elsif count_out(3) = '1' then
count_out <= count_out - X"0001";
end if;
end if;
end process;
end Behavioral;
```
3. **完整代码**:
这只是一个简化版的例子,完整的代码还需要添加错误检查和其他必要的条件,以及合适的注释来描述计数逻辑。
```vhdl
...
end Behavioral;
architecture Behavioral of FourBitReversibleCounter is
constant MAX_COUNT : integer := 2 ** 4 - 1; -- 计数器最大值
signal current_count_state : integer range 0 to MAX_COUNT; -- 当前计数值
begin
...
-- 更新当前计数状态
current_count_state <= (if reset = '1' or count_out(3) = '0' then 0 else current_count_state + signed(count_out(3)));
-- 输出计数值
count_out <= std_logic_vector(current_count_state);
...
end Behavioral;
```
阅读全文
相关推荐
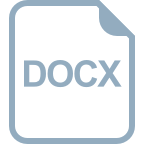
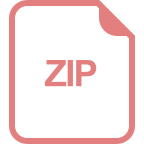
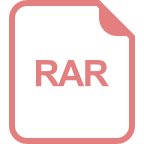
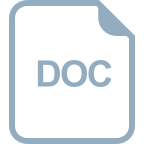

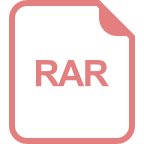
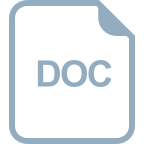
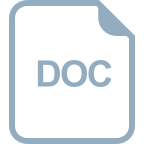
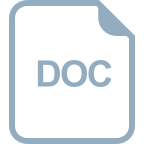








