# Indicates the change of voxel. mask = torch.ones(x.shape[0], device=x.device, dtype=torch.bool) mask[:-1] = ranks[1:] != ranks[:-1]这里的mask尺寸是多少?ranks[1:] != ranks[:-1]rank是什么含义?
时间: 2024-01-01 07:06:34 浏览: 22
这里的`mask`是一个布尔类型的一维张量,形状为`(x.shape[0],)`,其中`x.shape[0]`是特征张量`x`的长度。`mask`的作用是指示哪些特征张量属于同一体素。具体来说,如果`mask[i]`为True,则表示`x[i]`和`x[i+1]`不属于同一体素。在该代码中,`mask`的长度等于特征张量`x`的长度,因为它的每个元素对应于特征张量中的一个元素。
`ranks`是一个一维张量,形状与特征张量`x`相同,它包含了特征张量中每个元素所属的体素的索引。具体来说,`ranks[i]`是特征张量`x[i]`所属的体素的索引。在这个代码中,`ranks[1:] != ranks[:-1]`是一个布尔类型的一维张量,它用于比较相邻特征张量所属的体素索引是否相等。如果相邻特征张量所属的体素索引不相等,则相应的元素为True,否则为False。这个布尔类型张量的长度比特征张量`x`的长度少1,因为它比较的是相邻特征张量的体素索引。
相关问题
The dtype of attention mask (torch.int64) is not bool
The attention mask in Transformer models is typically a boolean tensor that indicates which tokens in the input sequence should be attended to and which ones should be ignored. If you're getting an error that says the dtype of the attention mask is not bool, it means that the tensor has a different data type (in this case, torch.int64) than expected.
To fix this, you can convert the attention mask tensor to bool using the `.bool()` method, like this:
```
attention_mask = attention_mask.bool()
```
This should ensure that the attention mask has the correct data type for the Transformer model.
X = data.iloc[:, :-1]
As an AI language model, I do not have access to the specific dataset that you are working with, so I can only provide a general explanation of this line of code.
The code "X = data.iloc[:, :-1]" is used to assign a subset of a dataset to a variable named "X".
The "data" variable is assumed to be a pandas DataFrame, which is a tabular data structure that can hold data of different types in columns.
The "iloc" method is used to select rows and columns of the DataFrame by their integer position.
The first parameter, ":", specifies that we want to select all rows of the DataFrame.
The second parameter, ":-1", specifies that we want to select all columns of the DataFrame except for the last one. The "-1" index indicates the last column, so ":-1" means "all columns up to but not including the last one".
Therefore, the "X" variable will contain all the rows of the DataFrame except for the last column. This is often done in machine learning tasks where the last column represents the target variable or output that we want to predict, and the remaining columns represent the input features.
相关推荐
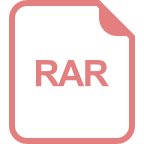
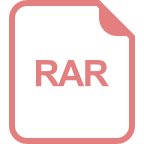
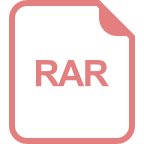










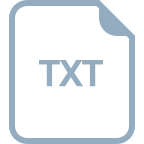
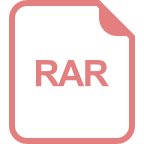
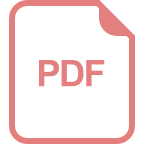