已知游戏中常见人物状态State抽象类,包括站立(Idle),行走(Run),攻击(Attack),死亡(Dead)等动作。在游戏中有角色hero类,拥有人物状态同时还拥有血量,攻击力,防御力等属性,有怪物类Monster,怪物有血量,攻击力,防御力,怪物类型等属性。 已知角色可以变身为怪物,现在main函数中,模拟某个角色在正常情况下以及在变身状态下的各种状态操作(输入1为正常hero,输入2则变身为monster)。 要求,使用抽象类及的基类指针实现。
时间: 2023-11-07 09:04:41 浏览: 129
下面是一个可能的实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 人物状态抽象类
class State {
public:
virtual void action() = 0; // 动作
};
// 站立状态
class Idle : public State {
public:
void action() {
cout << "Idle" << endl;
}
};
// 行走状态
class Run : public State {
public:
void action() {
cout << "Run" << endl;
}
};
// 攻击状态
class Attack : public State {
public:
void action() {
cout << "Attack" << endl;
}
};
// 死亡状态
class Dead : public State {
public:
void action() {
cout << "Dead" << endl;
}
};
// 人物基类
class Character {
protected:
State* state; // 当前状态
int hp; // 血量
int atk; // 攻击力
int def; // 防御力
public:
Character(int _hp, int _atk, int _def): state(new Idle), hp(_hp), atk(_atk), def(_def) {}
virtual ~Character() { delete state; }
void setState(State* _state) { state = _state; }
void action() { state->action(); }
virtual void printInfo() = 0; // 打印信息
};
// 英雄类
class Hero : public Character {
public:
Hero(int _hp, int _atk, int _def): Character(_hp, _atk, _def) {}
void printInfo() {
cout << "Hero: hp = " << hp << ", atk = " << atk << ", def = " << def << endl;
}
};
// 怪物类
class Monster : public Character {
private:
string type; // 怪物类型
public:
Monster(int _hp, int _atk, int _def, const string& _type): Character(_hp, _atk, _def), type(_type) {}
void printInfo() {
cout << "Monster: type = " << type << ", hp = " << hp << ", atk = " << atk << ", def = " << def << endl;
}
};
int main() {
Character* character = new Hero(100, 10, 5); // 初始为英雄
character->printInfo();
character->setState(new Run);
character->action();
character->setState(new Attack);
character->action();
character->setState(new Dead);
character->action();
delete character;
character = new Monster(50, 8, 3, "Goblin"); // 初始为怪物
character->printInfo();
character->setState(new Run);
character->action();
character->setState(new Attack);
character->action();
character->setState(new Dead);
character->action();
delete character;
return 0;
}
```
在这个实现中,State 是人物状态的抽象类,包括了站立、行走、攻击、死亡等动作,每个动作都有一个具体的实现类。Character 是人物的基类,包括了人物的状态、血量、攻击力、防御力等属性,以及操作状态和打印信息的接口。Hero 和 Monster 都是 Character 的子类,分别代表英雄和怪物,它们具有不同的打印信息。
在 main 函数中,首先创建一个 Hero 对象作为初始状态,然后模拟了一系列动作,最后销毁对象。接着按照同样的方式创建一个 Monster 对象,模拟一系列动作并销毁对象。在这个过程中,可以看到使用了抽象类及基类指针,可以方便地进行状态的切换和操作,从而实现了角色的状态转换。
阅读全文
相关推荐
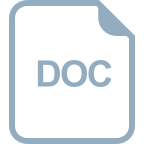
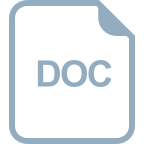
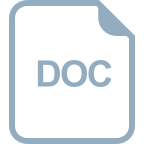





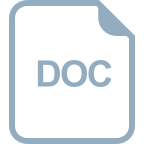
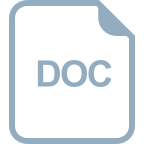
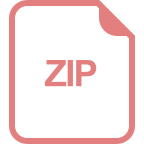
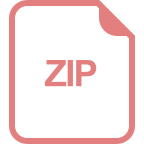
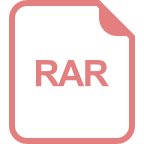
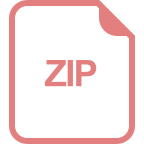
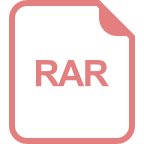