使用首次适应算法改写class MemoryManager: def __init__(self, allocator): self.allocator = allocator def allocate(self, process, request_size): memory_view = self.allocator.memory_view() '''这里是写的''' # Find the best fit block for the process best_fit_block = None best_fit_size = float('inf') for i in range(len(memory_view)): if memory_view[i] is None: # Check if the block is free block_size = 0 j = i while j < len(memory_view) and memory_view[j] is None: block_size += 1 j += 1 if block_size >= request_size and block_size < best_fit_size: best_fit_block = i best_fit_size = block_size if best_fit_block is not None: # Allocate memory for the process block_start = best_fit_block self.allocator.allocate_memory(block_start, request_size, process) else: # No suitable block found, allocation failed raise AssertionError('allocation failed')
时间: 2024-02-10 18:15:55 浏览: 129
可以将首次适应算法改写成以下代码:
```
class MemoryManager:
def __init__(self, allocator):
self.allocator = allocator
self.last_allocated = 0
def allocate(self, process, request_size):
memory_view = self.allocator.memory_view()
# Find the best fit block for the process
best_fit_block = None
best_fit_size = float('inf')
i = self.last_allocated
while i < len(memory_view):
if memory_view[i] is None: # Check if the block is free
block_size = 0
j = i
while j < len(memory_view) and memory_view[j] is None:
block_size += 1
j += 1
if block_size >= request_size and block_size < best_fit_size:
best_fit_block = i
best_fit_size = block_size
i += 1
# If no block is found from last allocated position to end then search from beginning
if best_fit_block is None:
i = 0
while i < self.last_allocated:
if memory_view[i] is None: # Check if the block is free
block_size = 0
j = i
while j < len(memory_view) and memory_view[j] is None:
block_size += 1
j += 1
if block_size >= request_size and block_size < best_fit_size:
best_fit_block = i
best_fit_size = block_size
i += 1
if best_fit_block is not None:
# Allocate memory for the process
block_start = best_fit_block
self.allocator.allocate_memory(block_start, request_size, process)
self.last_allocated = block_start + request_size
else:
# No suitable block found, allocation failed
raise AssertionError('allocation failed')
```
这里的改动在于在 `allocate` 方法中加入了 `self.last_allocated` 变量,它表示上一次分配内存时的位置,从这个位置开始向后搜索空闲块,避免在整个内存中搜索。如果在搜索到结尾仍然没有找到适合的块,则从头开始搜索。
阅读全文
相关推荐
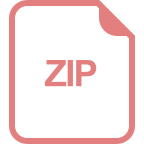
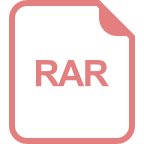
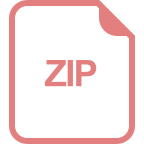














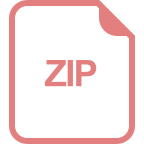
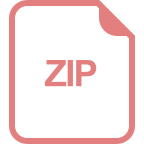