//------------Task 2. Create a list of students by reading from student_hobbies.txt and display the list. //You will also need to create a Student class, which has at least //two data fields: student name and an ArrayList of hobbies (String-type). System.out.println("\n------------ Task 2. Create a list of students by reading from student_hobbies.txt."); ArrayList <Student> studentList = new ArrayList<Student>(); studentList = readFile("student_hobbies_JU.txt"); for (Student s: studentList) { System.out.println(s); }
时间: 2024-02-28 10:57:10 浏览: 102
It looks like you have a good start to Task 2. However, in order for this code to work, you'll need to implement the `Student` class and the `readFile()` method.
Here's an example implementation of the `Student` class:
```java
import java.util.ArrayList;
public class Student {
private String name;
private ArrayList<String> hobbies;
public Student(String name, ArrayList<String> hobbies) {
this.name = name;
this.hobbies = hobbies;
}
public String getName() {
return name;
}
public ArrayList<String> getHobbies() {
return hobbies;
}
@Override
public String toString() {
return name + ": " + hobbies.toString();
}
}
```
This class has two data fields (`name` and `hobbies`) and a constructor that takes in a name and a list of hobbies. It also has getter methods for each data field and an overridden `toString()` method that returns a string representation of the `Student` object.
Here's an example implementation of the `readFile()` method:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
System.out.println("\n------------ Task 2. Create a list of students by reading from student_hobbies.txt.");
ArrayList<Student> studentList = readFile("student_hobbies_JU.txt");
for (Student s : studentList) {
System.out.println(s);
}
}
public static ArrayList<Student> readFile(String filename) {
ArrayList<Student> studentList = new ArrayList<>();
try (BufferedReader br = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = br.readLine()) != null) {
String[] parts = line.split(":");
String name = parts[0];
String[] hobbies = parts[1].split(",");
ArrayList<String> hobbyList = new ArrayList<>();
for (String h : hobbies) {
hobbyList.add(h.trim());
}
Student s = new Student(name, hobbyList);
studentList.add(s);
}
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
return studentList;
}
}
```
This code reads from the `student_hobbies_JU.txt` file (assuming it's in the same directory as the program) and creates a list of `Student` objects. It then displays each `Student` object using the `toString()` method we defined earlier.
I hope this helps! Let me know if you have any questions.
阅读全文
相关推荐
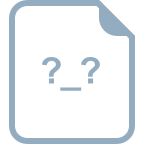
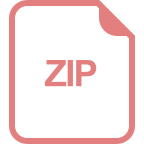
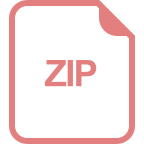




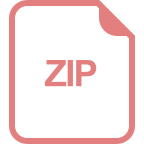
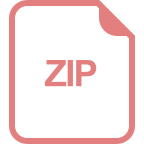
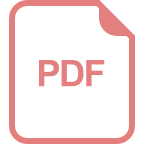
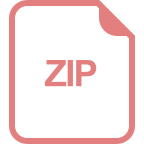





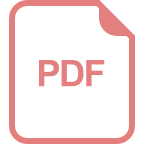