u = ((true-true.mean(0))*(pred-pred.mean(0))).sum(0) d = np.sqrt(((true-true.mean(0))**2*(pred-pred.mean(0))**2).sum(0)) return (u/d).mean(-1) 这段代码是什么意思
时间: 2024-04-06 11:28:12 浏览: 117
这段代码实现了计算 Pearson 相关系数。其中 true 和 pred 是两个数组,分别表示真实值和预测值。首先计算了每个特征的样本协方差 u,然后计算了每个特征的样本标准差 d,最后将 u/d 取平均得到 Pearson 相关系数。具体的实现步骤如下:
1. true 和 pred 分别减去各自的均值,并计算两者的乘积,得到样本协方差 u。
2. 对于每个特征,分别计算 true 和 pred 的方差乘积,并将所有特征的结果相加,再开方得到样本标准差 d。
3. 将 u/d 取平均,得到 Pearson 相关系数。
需要注意的是,这段代码中的计算都是针对每个特征进行的,因此需要在最后对所有特征的结果取平均。
相关问题
class FocalLoss(nn.Module): # Wraps focal loss around existing loss_fcn(), i.e. criteria = FocalLoss(nn.BCEWithLogitsLoss(), gamma=1.5) def __init__(self, loss_fcn, gamma=1.5, alpha=0.25): super(FocalLoss, self).__init__() self.loss_fcn = loss_fcn # must be nn.BCEWithLogitsLoss() self.gamma = gamma self.alpha = alpha self.reduction = loss_fcn.reduction self.loss_fcn.reduction = 'none' # required to apply FL to each element def forward(self, pred, true): loss = self.loss_fcn(pred, true) # p_t = torch.exp(-loss) # loss *= self.alpha * (1.000001 - p_t) ** self.gamma # non-zero power for gradient stability # TF implementation https://github.com/tensorflow/addons/blob/v0.7.1/tensorflow_addons/losses/focal_loss.py pred_prob = torch.sigmoid(pred) # prob from logits p_t = true * pred_prob + (1 - true) * (1 - pred_prob) alpha_factor = true * self.alpha + (1 - true) * (1 - self.alpha) modulating_factor = (1.0 - p_t) ** self.gamma loss *= alpha_factor * modulating_factor if self.reduction == 'mean': return loss.mean() elif self.reduction == 'sum': return loss.sum() else: # 'none' return loss
这个代码实现了一个 Focal Loss 损失函数,它是对二分类问题中的交叉熵损失函数的一种改进。它主要通过增加一个可调的超参数 $\gamma$,来调整难易样本的权重,从而解决类别不平衡问题。在代码中,它被实现为一个 PyTorch 模块,它的 forward 方法接受两个张量,即预测值和真实值,并返回计算出来的损失值。在实现中,它首先对传入的 loss_fcn 进行了封装,将其 reduction 属性设为 'none',然后在 forward 方法中使用封装后的 loss_fcn 计算出二分类交叉熵损失,接着使用预测值和真实值计算出概率值,根据概率值和超参数 $\gamma$ 计算出一个调制因子,最后将原始损失值乘以调制因子和一个可调的超参数 $\alpha$,从而得到最终的 Focal Loss 损失。
#预测因子(海温) #nino3.4赤道东太平洋(190-220,-5-5) a22=sst_djf.sel(lon=slice(190,220),lat=slice(5,-5)).mean(axis=1).mean(axis=1) a2=(a22-a22.mean())/a22.std() #赤道印度洋(50-80,-5-5) a33=sst_djf.sel(lon=slice(50,100),lat=slice(5,-5)).mean(axis=1).mean(axis=1) a3=(a33-a33.mean())/a33.std() #预测因子(环流场) #南欧(30-40,35-45) b11=hgt_djf.sel(lon=slice(30,40),lat=slice(45,35)).mean(axis=1).mean(axis=1) b1=(b11-b11.mean())/b11.std() #太平洋副高(120-180,-10-10) b22=hgt_djf.sel(lon=slice(120,180),lat=slice(10,-10)).mean(axis=1).mean(axis=1) b2=(b22-b22.mean())/b22.std() #印度洋(60-80,-10-10) b33=hgt_djf.sel(lon=slice(60,80),lat=slice(10,-10)).mean(axis=1).mean(axis=1) b3=(b33-b33.mean())/b33.std() x=np.vstack([(a2,a3,b1,b2,b3)]).T x2=np.vstack([(a2,b1)]).T y=pre_standard #多元线性回归 res=np.linalg.lstsq(x,y,rcond=None) n=res[0] ##各项系数 y_fit=(n.T*x).sum(axis=1) #拟合数据 res2=np.linalg.lstsq(x2,y,rcond=None) n2=res2[0] ##各项系数 y_fit2=(n2.T*x2).sum(axis=1) #拟合数据 #可视化 time=np.arange(1961,2017,1) fig = plt.figure(figsize=[16, 5]) ax = fig.add_subplot() ax.plot(time, y,marker='o', color='gray', markersize=5) ax.plot(time, y_fit,marker='*', color='b', markersize=5) ax.plot(time, y_fit2,marker='^', color='r', markersize=5) ax.set_title('model',fontsize=20,fontweight='bold') ax.set_xlabel('Time') ax.set_ylabel('Pre') plt.legend(['Source data','Fitted1','Fitted2'],frameon=False,loc='best') plt.show()选做剔除一年的交叉检验,独立试报
好的,我理解你的意思是要对这段代码进行交叉验证和独立测试,以评估模型的性能。交叉验证可以用来评估模型的泛化能力,独立测试可以用来测试模型的预测能力。以下是一个简单的交叉验证和独立测试的示例代码,仅供参考:
交叉验证:
```python
from sklearn.model_selection import KFold
# 将数据集分成 5 份,进行 5 折交叉验证
kf = KFold(n_splits=5, shuffle=True, random_state=1)
# 存储每次交叉验证的评估结果
scores = []
for train_idx, test_idx in kf.split(x):
# 划分训练集和验证集
x_train, x_valid = x[train_idx], x[test_idx]
y_train, y_valid = y[train_idx], y[test_idx]
# 训练模型
res = np.linalg.lstsq(x_train, y_train, rcond=None)
n = res[0]
# 在验证集上进行预测,计算评估指标
y_pred = (n.T * x_valid).sum(axis=1)
score = np.sqrt(((y_pred - y_valid) ** 2).mean())
scores.append(score)
# 计算交叉验证的平均评估指标
mean_score = np.mean(scores)
print('交叉验证 RMSE:', mean_score)
```
独立测试:
```python
# 划分训练集和测试集
train_size = 0.8
n_samples = x.shape[0]
n_train = int(train_size * n_samples)
x_train, x_test = x[:n_train], x[n_train:]
y_train, y_test = y[:n_train], y[n_train:]
# 训练模型
res = np.linalg.lstsq(x_train, y_train, rcond=None)
n = res[0]
# 在测试集上进行预测,计算评估指标
y_pred = (n.T * x_test).sum(axis=1)
test_score = np.sqrt(((y_pred - y_test) ** 2).mean())
print('独立测试 RMSE:', test_score)
```
需要注意的是,这里使用的是简单的线性回归模型,可能存在欠拟合或过拟合的问题,需要根据实际情况进行调整。另外,数据预处理和特征工程也是影响模型性能的重要因素,需要进行合理的处理。
阅读全文
相关推荐
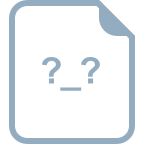
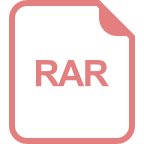
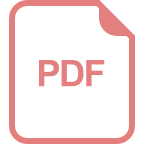














