有没有chatglm+neo4j的应用示例
时间: 2023-06-24 10:05:49 浏览: 223
ChatGLM是一个基于图神经网络的聊天机器人,而Neo4j是一个图数据库。将它们结合起来可以实现更加复杂的聊天机器人应用。以下是一个ChatGLM+Neo4j的应用示例:
假设我们要创建一个聊天机器人,它可以回答用户关于电影的问题。我们可以使用Neo4j存储电影信息和关系,然后使用ChatGLM作为聊天机器人引擎。
首先,我们需要将电影信息存储到Neo4j数据库中。我们可以使用Cypher语言来执行以下命令:
```
CREATE (:Movie {title: "The Shawshank Redemption", year: 1994, director: "Frank Darabont"})
CREATE (:Movie {title: "The Godfather", year: 1972, director: "Francis Ford Coppola"})
CREATE (:Movie {title: "The Dark Knight", year: 2008, director: "Christopher Nolan"})
```
这样我们就创建了三部电影的节点,并且每个节点都有标题、年份和导演属性。
接下来,我们需要将这些电影节点连接起来。我们可以使用以下命令:
```
MATCH (m1:Movie {title: "The Shawshank Redemption"}), (m2:Movie {title: "The Godfather"})
MERGE (m1)-[:SIMILAR_TO]->(m2)
MATCH (m1:Movie {title: "The Shawshank Redemption"}), (m2:Movie {title: "The Dark Knight"})
MERGE (m1)-[:SIMILAR_TO]->(m2)
```
这样我们就创建了两条关系,表示这三部电影之间的相似性。
现在我们已经将电影信息存储到Neo4j数据库中,我们可以使用ChatGLM作为聊天机器人引擎。ChatGLM可以使用Python编写,可以使用pytorch-geometric库来连接Neo4j数据库。以下是一个ChatGLM+Neo4j的Python示例代码:
```
import torch
from torch_geometric.nn import MessagePassing
from torch_geometric.utils import add_self_loops, degree
# Define the ChatGLM model
class ChatGLM(MessagePassing):
def __init__(self, in_channels, out_channels):
super(ChatGLM, self).__init__(aggr='add')
self.lin = torch.nn.Linear(in_channels, out_channels)
def forward(self, x, edge_index):
# Add self-loops to the adjacency matrix
edge_index, _ = add_self_loops(edge_index, num_nodes=x.size(0))
# Compute the degree of each node
row, col = edge_index
deg = degree(row, x.size(0), dtype=x.dtype)
# Apply the linear transformation
x = self.lin(x)
# Propagate the messages across the graph
return self.propagate(edge_index, x=x, deg=deg)
def message(self, x_j, deg):
# Normalize the messages by the degree of the source node
return x_j / deg.view(-1, 1)
# Connect to the Neo4j database
from neo4j import GraphDatabase
driver = GraphDatabase.driver(uri="bolt://localhost:7687", auth=("neo4j", "password"))
# Define a function to query the Neo4j database
def query_database(query):
with driver.session() as session:
result = session.run(query)
return [dict(record) for record in result]
# Define a function to get similar movies
def get_similar_movies(movie_title):
query = f"MATCH (m1:Movie {{title: '{movie_title}'}})-[:SIMILAR_TO]->(m2:Movie) RETURN m2.title"
result = query_database(query)
return [record['m2.title'] for record in result]
# Define a function to generate a response from the ChatGLM model
def generate_response(text):
# Convert the text to a vector using a pre-trained language model
vector = # code to convert text to vector using pre-trained language model
# Query the Neo4j database to get similar movies
similar_movies = get_similar_movies(text)
# Convert the similar movies to vectors using the same pre-trained language model
similar_vectors = # code to convert movies to vectors using pre-trained language model
# Compute the similarity between the input vector and the similar movie vectors
similarity = # code to compute similarity between input vector and movie vectors
# Compute the response using the ChatGLM model
response = # code to compute response using ChatGLM model
return response
# Test the ChatGLM+Neo4j model
text = "What movies are similar to The Shawshank Redemption?"
response = generate_response(text)
print(response)
```
这个示例代码演示了如何使用ChatGLM和Neo4j来实现一个电影聊天机器人。它可以接收用户的问题,并生成一个相应的回答。在这个例子中,我们使用Neo4j来存储电影信息和关系,并使用ChatGLM来处理自然语言输入和生成回答。
阅读全文
相关推荐
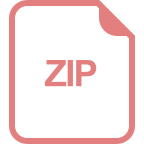
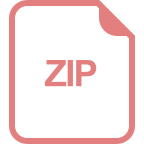
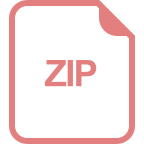















