devc++pta矩阵加法
时间: 2024-12-02 08:12:23 浏览: 19
Dev-C++ 是一款流行的 C++ 开发环境,它包含了一个名为 PTA(Personal Test Assistant,个人测试助手)的组件,通常用于编写和调试代码。对于矩阵加法这个数学操作,你可以用 C++ 编程语言来实现。以下是一个简单的例子,展示如何使用 Dev-C++ 实现两个矩阵的相加:
```cpp
#include <iostream>
using namespace std;
// 定义矩阵结构体
struct Matrix {
int rows, cols;
int data[100][100]; // 矩阵大小可以自定义,这里假设是10x10
};
// 矩阵加法函数
Matrix addMatrices(Matrix a, Matrix b) {
if (a.rows != b.rows || a.cols != b.cols) {
throw "Matrix dimensions do not match!";
}
Matrix result = {a.rows, a.cols};
for (int i = 0; i < a.rows; i++) {
for (int j = 0; j < a.cols; j++) {
result.data[i][j] = a.data[i][j] + b.data[i][j];
}
}
return result;
}
int main() {
Matrix m1 = {{1, 2}, {3, 4}};
Matrix m2 = {{5, 6}, {7, 8}};
try {
Matrix sum = addMatrices(m1, m2);
cout << "Matrix addition result:\n";
for (int i = 0; i < sum.rows; i++) {
for (int j = 0; j < sum.cols; j++) {
cout << sum.data[i][j] << "\t";
}
cout << endl;
}
} catch (const char* msg) {
cerr << msg << endl;
}
return 0;
}
```
在这个程序中,我们首先定义了一个 Matrix 结构体来表示矩阵,然后创建了 addMatrices 函数来进行矩阵加法。在主函数里,我们创建了两个矩阵并计算它们的和。
阅读全文
相关推荐
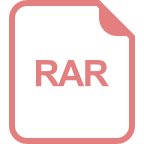
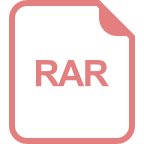















