用C++和opencv实现图像景深融合
时间: 2023-09-20 16:14:46 浏览: 164
图像景深融合是将多张不同焦距的图像融合成一幅具有较大景深的图像的过程。可以用C语言和OpenCV库来实现,具体实现步骤如下:
1. 读取不同焦距的图像并转换为灰度图像。
```C
Mat img1 = imread("image1.jpg", IMREAD_GRAYSCALE);
Mat img2 = imread("image2.jpg", IMREAD_GRAYSCALE);
// 确保两幅图像大小一致
resize(img1, img1, img2.size());
```
2. 计算两幅图像的深度图。
```C
Mat depth1, depth2;
// 分别计算两幅图像的深度图
// 可以使用Sobel算子、Laplacian算子等方法计算深度图
// 这里以Sobel算子为例
Sobel(img1, depth1, CV_8U, 1, 0);
Sobel(img2, depth2, CV_8U, 1, 0);
```
3. 计算两幅图像的权重图。
```C
Mat weight1, weight2;
// 根据深度图计算权重图
// 可以使用高斯函数、sigmoid函数等方法计算权重图
// 这里以高斯函数为例
GaussianBlur(depth1, depth1, Size(7, 7), 0);
GaussianBlur(depth2, depth2, Size(7, 7), 0);
normalize(depth1, depth1, 0, 1, NORM_MINMAX);
normalize(depth2, depth2, 0, 1, NORM_MINMAX);
weight1 = 1 - depth1;
weight2 = 1 - depth2;
```
4. 对两幅图像进行融合。
```C
Mat result;
// 将两幅图像根据权重进行融合
// 可以使用加权平均、拉普拉斯金字塔等方法进行融合
// 这里以加权平均方法为例
result = img1.mul(weight1) + img2.mul(weight2);
```
5. 显示融合后的图像。
```C
imshow("Result", result);
waitKey(0);
```
完整代码如下:
```C
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
// 读取不同焦距的图像并转换为灰度图像
Mat img1 = imread("image1.jpg", IMREAD_GRAYSCALE);
Mat img2 = imread("image2.jpg", IMREAD_GRAYSCALE);
// 确保两幅图像大小一致
resize(img1, img1, img2.size());
// 计算两幅图像的深度图
Mat depth1, depth2;
Sobel(img1, depth1, CV_8U, 1, 0);
Sobel(img2, depth2, CV_8U, 1, 0);
// 计算两幅图像的权重图
Mat weight1, weight2;
GaussianBlur(depth1, depth1, Size(7, 7), 0);
GaussianBlur(depth2, depth2, Size(7, 7), 0);
normalize(depth1, depth1, 0, 1, NORM_MINMAX);
normalize(depth2, depth2, 0, 1, NORM_MINMAX);
weight1 = 1 - depth1;
weight2 = 1 - depth2;
// 对两幅图像进行融合
Mat result;
result = img1.mul(weight1) + img2.mul(weight2);
// 显示融合后的图像
imshow("Result", result);
waitKey(0);
return 0;
}
```
注意:上述代码中的方法只是其中一种实现方式,可以根据具体应用场景选择不同的方法。此外,深度图和权重图的计算方法也可以根据具体应用场景进行调整。
相关推荐
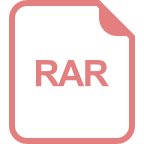
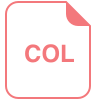
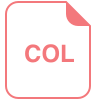
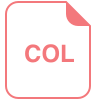
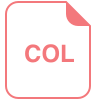
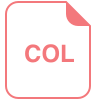









