For this problem, we'll round an int value up to the next multiple of 10 if its rightmost digit is 5 or more, so 15 rounds up to 20. Alternately, round down to the previous multiple of 10 if its rightmost digit is less than 5, so 12 rounds down to 10. Given 3 ints, a b c, return the sum of their rounded values. To avoid code repetition, write a separate helper "def round10(num):" and call it 3 times. Write the helper entirely below and at the same indent level as round_sum().
时间: 2023-12-21 07:03:47 浏览: 63
Here's the implementation of the `round10(num)` helper function:
```python
def round10(num):
if num % 10 >= 5:
return (num // 10 + 1) * 10
else:
return (num // 10) * 10
```
And here's how you can use it in the `round_sum()` function to solve the problem:
```python
def round_sum(a, b, c):
return round10(a) + round10(b) + round10(c)
```
In this implementation, we're calling the `round10()` function three times with the input values `a`, `b`, and `c`, and then returning the sum of their rounded values. The `round10()` function takes an integer as input and returns the nearest multiple of 10 based on its rightmost digit.
相关问题
rightmost digit c语言
rightmost digit是指一个数的最右边的数字,通常在计算机编程中用来进行数值运算。在C语言中,可以使用取模运算(%)来获取一个数的最右边的数字。例如,对于整数n,n%10的结果就是n的最右边的数字。
A block of 64 contiguous LAPs is reserved for inquiry operations; one LAP common to all devices is reserved for general inquiry, the remaining 63 LAPs are reserved for dedicated inquiry of specific classes of devices (see Assigned Numbers). The same LAP values are used regardless of the contents of UAP and NAP. Consequently, none of these LAPs can be part of a user BD_ADDR. The reserved LAP addresses are 0x9E8B00 to 0x9E8B3F. The general inquiry LAP is 0x9E8B33. All addresses have the LSB at the rightmost position, hexadecimal notation. The default check initialization (DCI) is used as the UAP whenever one of the reserved LAP addresses is used. The DCI is defined to be 0x00 (hexadecimal).
有一组64个连续的LAP保留用于查询操作;其中一个LAP是所有设备共用的,用于通用查询,剩下的63个LAP保留用于针对特定设备类别的专用查询(请参阅分配的编号)。无论UAP和NAP的内容如何,都使用相同的LAP值。因此,这些LAP都不能成为用户BD_ADDR的一部分。保留的LAP地址为0x9E8B00至0x9E8B3F。通用查询LAP为0x9E8B33。所有地址的最低有效位位于最右边的位置,使用十六进制表示。当使用保留的LAP地址之一时,使用默认检查初始化(DCI)作为UAP。DCI被定义为0x00(十六进制)。
阅读全文
相关推荐
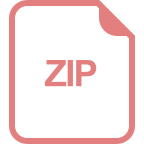
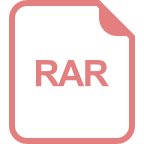
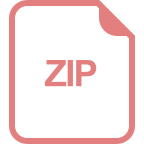
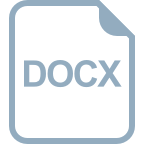
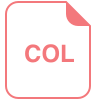












