爬取网站http://tipdm.com/的首页展示的产品信息内容,并将数据存储至本地csv文件中。
时间: 2023-12-21 16:04:26 浏览: 204
好的,我们可以使用 Python 中的 `requests` 和 `BeautifulSoup` 库来实现该功能。先使用 `requests` 库发送 GET 请求,然后使用 `BeautifulSoup` 库解析 HTML 页面,提取出我们需要的数据,最后将数据存储为 CSV 文件。
请先在命令行中输入以下命令安装 `requests` 和 `BeautifulSoup` 库:
```
pip install requests beautifulsoup4
```
安装完成后,可以使用以下 Python 代码实现你的需求:
```python
import csv
import requests
from bs4 import BeautifulSoup
url = 'http://tipdm.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
product_list = []
# 找到所有产品信息所在的 div 标签
div_list = soup.find_all('div', class_='product-item')
# 遍历每个产品信息 div 标签
for div in div_list:
# 提取产品名称和价格
name = div.find('div', class_='product-title').text.strip()
price = div.find('div', class_='product-price').text.strip()
# 将提取的信息添加到 product_list 列表中
product_list.append([name, price])
# 将产品信息保存到 CSV 文件中
with open('products.csv', 'w', newline='', encoding='utf-8-sig') as f:
writer = csv.writer(f)
writer.writerow(['名称', '价格'])
writer.writerows(product_list)
```
在这个代码中,我们先使用 `requests` 库中的 `get()` 方法发送一个 GET 请求,获取 http://tipdm.com/ 的首页页面信息。
然后,我们使用 `BeautifulSoup` 库解析 HTML 页面,并找到所有产品信息所在的 `div` 标签。遍历每个产品信息 `div` 标签,提取产品名称和价格,并将提取的信息添加到 `product_list` 列表中。
最后,我们使用 `csv` 库将产品信息保存到 CSV 文件中。在 `with open()` 语句中指定了文件名为 `products.csv`,打开文件的模式为写入模式,编码格式为 `utf-8-sig`(用于解决中文乱码问题)。使用 `csv.writer()` 方法创建一个 `writer` 对象,并使用 `writerow()` 和 `writerows()` 方法将产品信息写入 CSV 文件中。
注意,本示例代码中的 URL 是一个有效的链接,但并不保证在未来一直有效。如果需要爬取其他网站或页面,请将 `url` 变量替换为相应的链接。
阅读全文
相关推荐
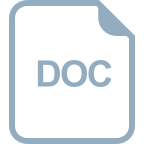
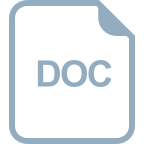
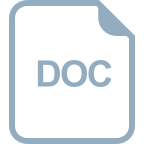









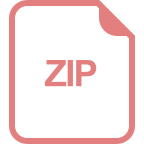



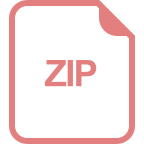
