如何用C语言实现单链表中第n个节点与第n+1个节点的元素互换操作?
时间: 2024-10-29 11:10:19 浏览: 25
在C语言中,实现单链表中两个相邻节点元素的互换通常需要遍历链表找到目标位置,然后临时存储数据,交换节点值,最后释放临时存储空间。这里是一个简单的步骤:
1. 定义结构体 `Node` 表示链表节点,包含 `data` 存储节点值,`next` 指向下一个节点。
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. 编写函数 `swapNodes(Node** head, int n)` 来完成操作,输入是头指针 `head` 和要交换的位置 `n`。
```c
void swapNodes(Node** head, int n) {
if (!(*head) || n <= 0) return; // 链表为空或n无效
Node* current = *head;
Node* temp = NULL;
// 遍历到第n个节点
for (int i = 1; i < n && current != NULL; ++i) {
current = current->next;
}
// 如果找到了n个节点,继续找到第n+1个
if (current == NULL || current->next == NULL) return;
temp = current->next; // 保存第n+1个节点
// 交换当前节点和第n+1个节点的数据
current->data = temp->data;
temp->data = current->next->data; // 交换下一个是直接赋值,因为已经保存了目标数据
current->next = temp->next; // 更新当前节点的下一个指向第n+2个节点
// 如果还有下一个小于n+2的节点,将其移到temp位置
if (temp->next != NULL) temp->next = current;
}
```
阅读全文
相关推荐
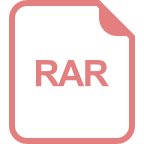
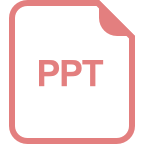
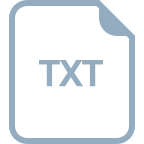




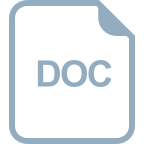
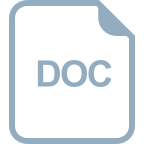
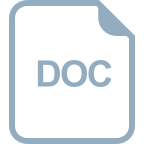
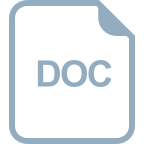







