c# 读取 doc文档 的表格
时间: 2024-09-12 09:13:04 浏览: 46
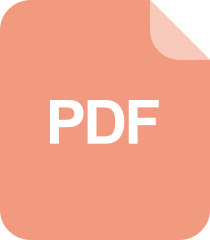
C#编程读取文档Doc、Docx及Pdf内容的方法
在C#中,读取Word文档(.doc格式通常由Microsoft Word创建,但现在更常见的可能是.docx格式,这是Office 2007及以后版本使用的格式)中的表格数据,可以使用第三方库如`Microsoft.Office.Interop.Word`(对于较旧的.doc文件)或`DocumentFormat.OpenXml`(用于.docx)。以下是使用这两个库的基本步骤:
1. 对于`.doc`文件(需引用Interop套件):
```csharp
using Microsoft.Office.Interop.Word;
// 加载Word应用程序
Application wordApp = new Application();
Document wordDoc = wordApp.Documents.Open("path_to_your_file.doc");
// 找到第一个表格
Table firstTable = wordDoc.Tables[1];
// 遍历行和列获取数据
foreach (Row row in firstTable.Rows)
{
foreach (Cell cell in row.Cells)
{
string data = cell.Range.Text; // 获取单元格内容
Console.WriteLine(data);
}
}
// 关闭文档并退出Word应用程序
wordDoc.Close();
wordApp.Quit();
```
2. 对于`.docx`文件(需引用OpenXML套件):
```csharp
using DocumentFormat.OpenXml.Packaging;
using DocumentFormat.OpenXml.Wordprocessing;
// 加载Word2007+文档
using (var package = WordprocessingDocument.Open("path_to_your_file.docx", true))
{
// 查找第一个表格
var mainPart = package.MainDocumentPart;
var body = mainPart.Document.Body;
var tables = body.Descendants<Table>();
// 选择第一个表格
Table firstTable = tables.FirstOrDefault();
if (firstTable != null)
{
foreach (TableRow tableRow in firstTable.Rows)
{
foreach (TableCell tableCell in tableRow.Cells)
{
string data = tableCell.InnerText; // 获取单元格内容
Console.WriteLine(data);
}
}
}
// 关闭流
package.Close();
}
```
请注意,这两种方法都需要安装对应的库,并且可能会受限于权限问题,如果文件是受密码保护的,需要处理相应的验证流程。
阅读全文
相关推荐
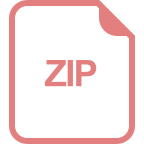
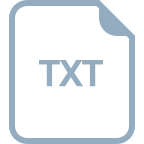















