请输入年月日,判断是今年的第几天
时间: 2024-06-13 21:04:04 浏览: 67
这个程序可以根据输入的年月日,判断是今年的第几天。具体实现方法是先根据输入的月份和日期,计算出该日期是该年的第几天,然后再根据闰年和平年的规则进行修正。下面是一个类似的Python程序实现:
```
# 输入年月日,判断是今年的第几天
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
# 构建闰年和平年的月份天数列表
leap_year_days = [0, 31, 60, 91, 121, 152, 182, 213, 244, 274, 305, 335]
common_year_days = [0, 31, 59, 90, 120, 151,181, 212, 243, 273, 304, 334]
# 判断是否为闰年
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
days = leap_year_days[month - 1] + day
else:
days = common_year_days[month - 1] + day
# 修正闰年和平年的天数
if month > 2 and ((year % 4 == 0 and year % 100 ! 0) or year % 400 == 0):
days += 1
print("这是今年的第%d天。 % days)
```
相关问题
用计算机元表示输入年月日判断是第几天,根据输入的年月日判断是本年的第几天(数组)...
好的,我可以为您提供一个简单的 Python 代码来实现这个功能:
```python
# 定义每个月的天数
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
# 获取用户输入的年月日
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
# 判断是否为闰年,闰年2月有29天
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
days_in_month[1] = 29
# 计算天数
day_count = sum(days_in_month[:month-1]) + day
# 输出结果
print("这是第%d天。" % day_count)
```
这个代码首先定义了每个月的天数,在用户输入年月日后,判断是否为闰年(闰年2月有29天),然后根据输入的月份和日期计算出天数并输出结果。
注意:这个代码中的年份必须是公元后的年份。如果您需要处理公元前的年份,需要对闰年的判断进行修改。
c语言输入年月日判断第几天
可以按照以下步骤来实现:
1. 从控制台或文件输入年月日。
2. 根据输入的年份,计算出该年是否为闰年。
- 如果该年能被4整除但不能被100整除,或者能被400整除,则为闰年。
3. 根据输入的月份和是否为闰年,计算出该月份的天数。
4. 将输入的天数加上前面所有月份的天数,即可得到该日期是该年的第几天。
下面是一个示例代码:
```c
#include <stdio.h>
int main() {
int year, month, day;
int days[] = {31,28,31,30,31,30,31,31,30,31,30,31}; // 每个月的天数,2月份默认为28天
int totalDays = 0; // 该日期是该年的第几天
int i;
// 输入年月日
printf("请输入年月日,格式为yyyy-mm-dd:");
scanf("%d-%d-%d", &year, &month, &day);
// 判断闰年
if ((year%4==0 && year%100!=0) || year%400==0) {
days[1] = 29; // 闰年2月份为29天
}
// 计算天数
for (i = 0; i < month - 1; i++) {
totalDays += days[i];
}
totalDays += day;
// 输出结果
printf("%d年%d月%d日是该年的第%d天\n", year, month, day, totalDays);
return 0;
}
```
注意:这段代码只是一个示例,可能存在一些未考虑的情况,需要根据具体情况进行调整。
阅读全文
相关推荐
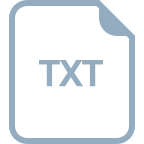













