c语言,输入年月日,判断第几天
时间: 2024-10-18 19:03:18 浏览: 19
在C语言中,判断给定日期是当年的第几天,需要考虑闰年和平年的规则。首先你需要让用户输入年、月和日,然后按照以下步骤计算:
1. 确定是否是闰年:如果该年份能被4整除但不能被100整除,或者是可以被400整除的,则该年是闰年。
2. 计算每个月的天数:
- 1月和2月在非闰年有31天,闰年有29天。
- 3月到11月都是30天。
- 12月无论平闰都有31天。
3. 对于用户输入的日期,从1月开始逐个月累加天数,同时注意调整闰年的2月份天数。
下面是一个简单的示例代码片段,用于演示这个过程:
```c
#include <stdio.h>
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int days_in_month(int month, int year) {
if (month <= 2 && is_leap_year(year)) {
return 29;
} else if (month > 2) {
switch (month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
return 31;
default:
return 30;
}
}
return 28;
}
int main() {
int year, month, day;
printf("请输入年月日(例如:2023 2 28): ");
scanf("%d %d %d", &year, &month, &day);
int total_days = 0;
for (int i = 1; i < month; i++) {
total_days += days_in_month(i, year);
}
total_days += day;
printf("给定日期%d/%d/%d是当年的第%d天。\n", day, month, year, total_days);
return 0;
}
```
阅读全文
相关推荐
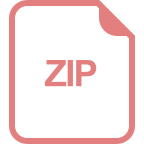
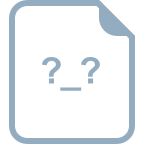
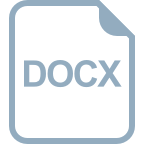



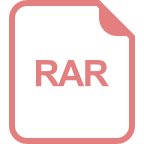
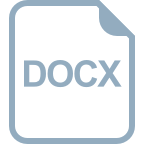










