在二叉搜索树中插入节点后如何进行平衡操作以维护树的平衡性?请提供Java实现的示例代码。
时间: 2024-11-05 22:22:23 浏览: 25
二叉搜索树在插入节点后可能会失去平衡,导致性能下降。为了保持树的平衡性,可以采用如AVL树或红黑树这类自平衡二叉搜索树。下面是一个Java示例代码,展示如何在插入节点后通过左旋和右旋操作来调整AVL树的平衡性:
参考资源链接:[掌握数据结构:树的详解与Java实现及应用场景](https://wenku.csdn.net/doc/4aew768321?spm=1055.2569.3001.10343)
```java
class AVLTree {
private TreeNode root;
private int height(TreeNode node) {
if (node == null) {
return -1;
}
return Math.max(height(node.left), height(node.right)) + 1;
}
private TreeNode rightRotate(TreeNode y) {
TreeNode x = y.left;
TreeNode T2 = x.right;
// Perform rotation
x.right = y;
y.left = T2;
// Update heights
y.height = Math.max(height(y.left), height(y.right)) + 1;
x.height = Math.max(height(x.left), height(x.right)) + 1;
// Return new root
return x;
}
private TreeNode leftRotate(TreeNode x) {
TreeNode y = x.right;
TreeNode T2 = y.left;
// Perform rotation
y.left = x;
x.right = T2;
// Update heights
x.height = Math.max(height(x.left), height(x.right)) + 1;
y.height = Math.max(height(y.left), height(y.right)) + 1;
// Return new root
return y;
}
// Update the height of the ancestor node
private void updateHeight(TreeNode node) {
node.height = 1 + Math.max(height(node.left), height(node.right));
}
// Get Balance factor of node N
private int getBalance(TreeNode node) {
if (node == null) {
return 0;
}
return height(node.left) - height(node.right);
}
public void insert(int key) {
root = insertRec(root, key);
}
private TreeNode insertRec(TreeNode root, int key) {
// 1. Perform the normal BST insertion
if (root == null) {
return (new TreeNode(key));
}
if (key < root.val) {
root.left = insertRec(root.left, key);
} else if (key > root.val) {
root.right = insertRec(root.right, key);
} else {
// Duplicate keys not allowed
return root;
}
// 2. Update height of this ancestor node
updateHeight(root);
// 3. Get the balance factor of this ancestor node to check whether this node became unbalanced
int balance = getBalance(root);
// If this node becomes unbalanced, then there are 4 cases
// Left Left Case
if (balance > 1 && key < root.left.val) {
return rightRotate(root);
}
// Right Right Case
if (balance < -1 && key > root.right.val) {
return leftRotate(root);
}
// Left Right Case
if (balance > 1 && key > root.left.val) {
root.left = leftRotate(root.left);
return rightRotate(root);
}
// Right Left Case
if (balance < -1 && key < root.right.val) {
root.right = rightRotate(root.right);
return leftRotate(root);
}
// return the (unchanged) node pointer
return root;
}
}
```
在此示例代码中,AVL树的节点插入操作后进行了高度更新和平衡因子检查,如果发现不平衡则根据不同的情况进行左旋、右旋或复合旋转操作,以确保树的平衡性。此技术细节可帮助你实现一个平衡的二叉搜索树,从而在实际应用中优化搜索和检索的效率。如果你需要更全面了解树结构的应用和优化,推荐参阅《掌握数据结构:树的详解与Java实现及应用场景》一书,它提供了更多的数据结构知识、Java实现和应用场景分析,有助于你在树结构及其应用方面达到更高的水平。
参考资源链接:[掌握数据结构:树的详解与Java实现及应用场景](https://wenku.csdn.net/doc/4aew768321?spm=1055.2569.3001.10343)
阅读全文
相关推荐
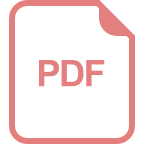
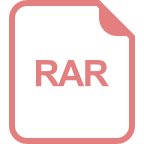
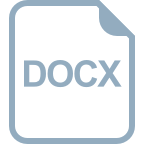

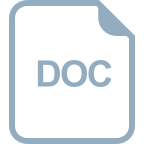
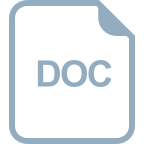
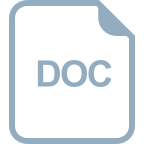
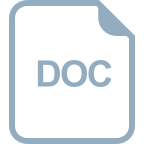
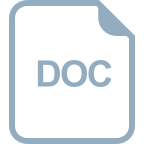
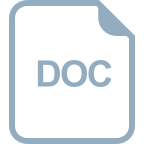
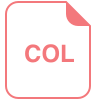
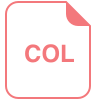
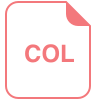
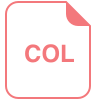
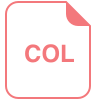
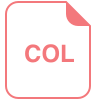
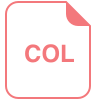
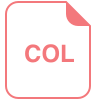

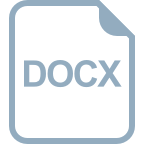