输入一个月份,输出该月有多少天,考虑闰年,编写一个c语言
时间: 2024-11-15 15:34:06 浏览: 49
在C语言中,为了计算一年中任意月份的天数,特别是考虑到闰年,我们可以创建一个函数来处理这个任务。这里是一个简单的实现,包括闰年的规则(每4年一闰,世纪年需要是400的倍数才是闰年):
```c
#include <stdio.h>
#include <stdbool.h>
bool is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int days_in_month(int month, int year) {
switch(month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return 31;
case 4:
case 6:
case 9:
case 11:
return 30;
case 2:
// 考虑闰年
if (is_leap_year(year)) {
return 29;
}
return 28;
default:
// 确保输入的有效性
return -1; // 或者抛出错误
}
}
int main() {
int month, year;
printf("请输入月份(1-12)和年份: ");
scanf("%d %d", &month, &year);
if (month >= 1 && month <= 12) { // 检查月份有效性
int days = days_in_month(month, year);
if (days != -1) {
printf("月份 %d 的天数为:%d\n", month, days);
} else {
printf("无效的月份输入.\n");
}
} else {
printf("无效的月份输入.\n");
}
return 0;
}
```
在这个程序中,`days_in_month`函数通过`switch`语句处理每个月的天数,并根据闰年规则调整二月的天数。主函数接受用户输入的月份和年份,调用`days_in_month`函数并输出结果。
阅读全文
相关推荐
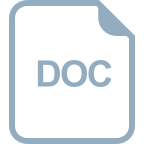
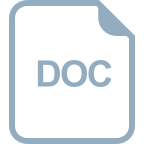
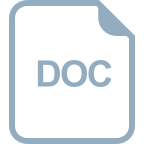
















