加法密码加密函数:根据加密公式,写一个Java程序实现明文到密文和密文到明文的转换。
时间: 2024-09-26 18:05:53 浏览: 46
加法密码(Additive Cipher),也称为简单替换密码的一种变体,是一种基于数学加法操作的加密技术。在这种方法中,每个明文字母通过与一个固定的密钥字母相加(模26取余)来进行加密。以下是使用Java实现一个简单的加法密码加密和解密函数的示例:
```java
public class AdditiveCipher {
private char[] alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ".toCharArray(); // 字母表
// 加密函数
public String encrypt(String plaintext, char key) {
StringBuilder ciphertext = new StringBuilder();
for (char c : plaintext.toCharArray()) {
if (Character.isLetter(c)) { // 只处理大写字母
int encryptedIndex = (alphabet.indexOf(c) + key - 'A') % 26;
ciphertext.append(alphabet[encryptedIndex]);
} else {
ciphertext.append(c); // 非字母字符不变
}
}
return ciphertext.toString();
}
// 解密函数
public String decrypt(String ciphertext, char key) {
return encrypt(ciphertext, key);
}
public static void main(String[] args) {
AdditiveCipher cipher = new AdditiveCipher();
String plaintext = "Hello, World!";
char key = 'K'; // 密钥
System.out.println("Original Text: " + plaintext);
String encrypted = cipher.encrypt(plaintext, key);
System.out.println("Encrypted Text: " + encrypted);
String decrypted = cipher.decrypt(encrypted, key);
System.out.println("Decrypted Text: " + decrypted);
}
}
```
在这个例子中,`encrypt`函数接受明文和密钥作为输入,`decrypt`函数则是其逆过程。注意,这个实现仅适用于英文大写字母,其他字符保持原样。
相关推荐
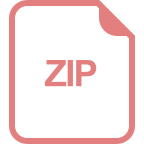
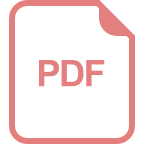
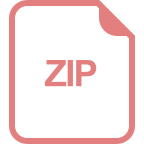















