在一个.cpp文件设计了一个函数并将其.h文件生成,如何用一个test.cpp文件对其进行测试
时间: 2024-04-15 07:27:32 浏览: 122
你可以按照以下步骤来测试你的函数:
1. 在test.cpp文件中包含你的函数的头文件。使用`#include "your_function.h"`来引入头文件。
2. 创建一个测试函数,在该函数中调用你的函数,并打印或者处理返回结果。例如:
```cpp
#include "your_function.h"
#include <iostream>
int main() {
// 调用你的函数进行测试
int result = your_function(); // 假设你的函数返回一个整数
// 打印结果
std::cout << "Result: " << result << std::endl;
return 0;
}
```
3. 编译并运行test.cpp文件。你可以使用C++编译器(如g++)来编译这个文件。在命令行中执行以下命令:
```
g++ test.cpp -o test
```
这将生成一个可执行文件`test`。
4. 运行生成的可执行文件:
```
./test
```
你将看到测试结果被打印在控制台上。
这是一个基本的方法来测试你的函数。根据你的具体需求,你可能需要编写更多的测试用例来覆盖不同的情况。
相关问题
在catch.cpp测试框架中,存在3个测试文件test_mutex.cpp、test_sem.cpp、test_task.cpp,如何把test_main.cpp文件中的函数或者变量,给到这些测试中使用?
在`catch.cpp`测试框架中,通常使用模块化的设计思想,每个单独的测试文件如`test_mutex.cpp`、`test_sem.cpp`和`test_task.cpp`关注特定的功能或模块。如果要在`test_main.cpp`中定义的函数或变量需要供这些测试文件使用,可以采用以下几种方式:
1. 公开全局变量:在`test_main.cpp`中声明并初始化那些需要共享的变量,然后在`CMakeLists.txt`中设置为公共可见。其他测试文件可以直接引用这些全局变量。
```cpp
// test_main.cpp
extern std::mutex globalMutex;
// 然后在test_mutex.cpp中使用
void someFunction() {
std::lock_guard<std::mutex> lock(globalMutex);
// ...
}
```
2. 函数原型和实现分离:在`test_main.cpp`中提供函数的声明(无实现),然后在相应的测试文件里包含该头文件并实现它。
```cpp
// test_main.cpp
void initGlobalFunctions();
// test_mutex.cpp
#include "test_main.cpp" // 包含函数声明
int main() {
initGlobalFunctions();
// ...
}
// 在test_main.cpp中实现函数
void initGlobalFunctions() {
// 初始化函数...
}
```
3. 使用依赖注入:在`test_main.cpp`中创建一个专门的类或结构体,并提供构造函数或成员函数来设置共享资源。测试文件通过实例化这个类来访问这些功能。
```cpp
// test_main.cpp
class TestContext {
public:
explicit TestContext(std::mutex& mutex) : myMutex(mutex) {}
void someFunction() { ... }
private:
std::mutex& myMutex;
};
// test_mutex.cpp
TestContext context(globalMutex);
context.someFunction();
```
无论哪种方法,都建议避免全局状态过多,保持良好的封装性和单一职责原则。同时记得在每个测试运行结束后清理资源,以防止潜在的内存泄漏。
存在3个测试文件test_mutex.cpp、test_sem.cpp、test_task.cpp,如何把test_main.cpp文件中的函数或者变量,给到这些测试中使用?
通常在C++中,为了在多个测试文件中复用test_main.cpp的功能,如函数或变量,你可以采用以下几种方式:
1. **公共头文件**:创建一个名为common.h或者util.h这样的头文件,包含你需要在所有测试文件中使用的函数声明或者变量定义。然后在test_main.cpp里包含这个头文件,并定义相关的功能。
```cpp
// common.h
#ifndef COMMON_H
#define COMMON_H
#include <iostream>
// 函数声明或变量定义
void someFunction();
extern int globalVariable;
// ... 其他内容 ...
#endif // COMMON_H
// test_main.cpp
#include "common.h"
void someFunction() {
// 函数体...
}
int main() {
// 初始化全局变量
globalVariable = 0;
// 进行其他初始化...
// 测试代码调用公共函数
someFunction();
return 0;
}
```
2. **测试框架支持**:如果你正在使用像Google Test (gtest)这样的测试框架,可以将测试用例类作为公共部分,在test_main.cpp中实例化并注册它。这样每个测试文件只需包含该测试类即可。
```cpp
// test_case.h
class TestCase {
public:
void runSomeTest();
};
// test_main.cpp
int main(int argc, char **argv) {
::testing::InitGoogleTest(&argc, argv);
auto test_case = new TestCase();
RUN_ALL_TESTS(); // 使用Google Test运行所有测试
delete test_case;
return 0;
}
// test_mutex.cpp, test_sem.cpp, test_task.cpp
#include "test_case.h"
TEST_F(TestCase, MutexTest) { /* 测试代码 */ }
TEST_F(TestCase, SemaphoresTest) { /* 测试代码 */ }
TEST_F(TestCase, TaskTest) { /* 测试代码 */ }
```
阅读全文
相关推荐





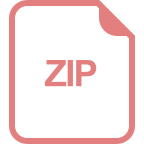
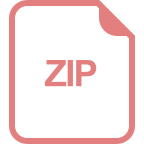
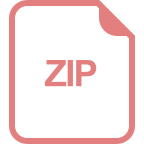




