在pycharm中,用高斯-赛德尔迭代法,求解如下方程组 3x₁+5x₂-6x₃=1 5x₁-7x₂+5x₃=-3 7x₁+11x₂-8x₃=10
时间: 2024-10-17 21:10:16 浏览: 16
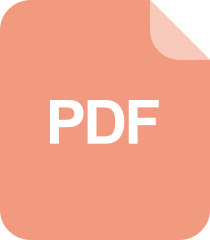
在Python3.74+PyCharm2020.1 x64中安装使用Kivy的详细教程
在PyCharm中,高斯-赛德尔迭代法(Gauss-Seidel method)通常用于求解线性代数中的大型稀疏矩阵方程组。这是一种迭代算法,通过逐步更新每个未知数的值,直到达到收敛或者满足预设的停止条件。对于给定的方程组,我们可以将其表示为以下形式:
\[
\begin{cases}
3x_1 + 5x_2 - 6x_3 = 1 \\
5x_1 - 7x_2 + 5x_3 = -3 \\
7x_1 + 11x_2 - 8x_3 = 10
\end{cases}
\]
为了在PyCharm中实现这个算法,你需要将方程组转换成矩阵形式,并创建一个迭代函数。首先,我们需要导入必要的数学库(如numpy),然后定义系数矩阵A和常数向量b。以下是简化版的步骤:
1. 导入所需库:
```python
import numpy as np
```
2. 定义系数矩阵A和常数向量b:
```python
A = np.array([[3, 5, -6], [5, -7, 5], [7, 11, -8]])
b = np.array([1, -3, 10])
```
3. 初始化未知数向量x(初始猜测)和迭代次数:
```python
tolerance = 1e-6
max_iterations = 1000
x_guess = np.zeros_like(A[:, 0]) # 初始猜测全为0
```
4. 实现高斯-赛德尔迭代:
```python
def gauss_seidel(A, b, x_guess, tolerance, max_iterations):
for _ in range(max_iterations):
x_new = np.copy(x_guess)
x_new[0] = (b[0] - A[0, 2]*x_guess[2]) / A[0, 0]
x_new[1] = (b[1] - A[1, 0]*x_guess[0] - A[1, 2]*x_guess[2]) / A[1, 1]
x_new[2] = (b[2] - A[2, 0]*x_guess[0] - A[2, 1]*x_guess[1]) / A[2, 2]
if np.linalg.norm(x_guess - x_new) < tolerance:
break
x_guess = x_new
return x_new
```
5. 调用函数并打印结果:
```python
solution = gauss_seidel(A, b, x_guess, tolerance, max_iterations)
print(f"Solutions after {max_iterations} iterations: {solution}")
```
阅读全文
相关推荐
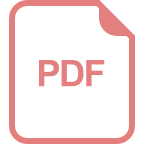
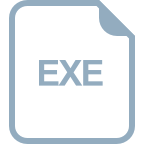

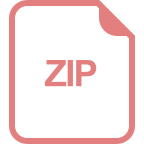
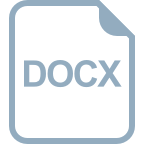
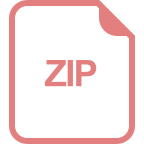
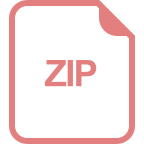
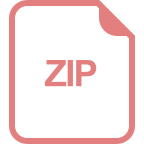
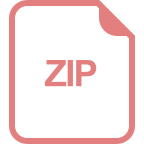
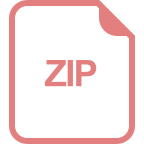
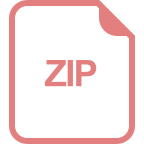
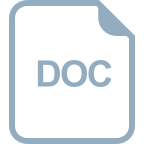
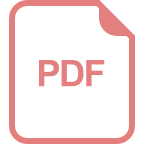
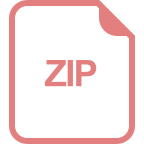