this.jdbcTemplate.update
时间: 2024-06-16 07:07:05 浏览: 182
this.jdbcTemplate.update是Spring框架中的一个方法,用于执行SQL更新操作。它是JdbcTemplate类的一个方法,JdbcTemplate是Spring提供的一个用于简化数据库操作的工具类。
该方法的作用是执行SQL语句,可以是INSERT、UPDATE或DELETE语句,用于对数据库进行增、删、改操作。它接收两个参数:SQL语句和参数值。SQL语句可以使用占位符来表示参数值,参数值可以通过数组或Map来传递。
该方法会返回一个整数值,表示受影响的行数,即执行SQL语句后数据库中受影响的行数。
使用this.jdbcTemplate.update方法可以方便地执行SQL更新操作,避免了手动创建连接、编写try-catch代码块等繁琐的操作。
相关问题
jdbcTemplate.batchUpdate() insert语句的示例
Sure! Here's an example of using `jdbcTemplate.batchUpdate()` for inserting data into a database table:
```java
public void batchInsert(List<User> users) {
String sql = "INSERT INTO users (id, name, age) VALUES (?, ?, ?)";
jdbcTemplate.batchUpdate(sql, new BatchPreparedStatementSetter() {
@Override
public void setValues(PreparedStatement ps, int i) throws SQLException {
User user = users.get(i);
ps.setInt(1, user.getId());
ps.setString(2, user.getName());
ps.setInt(3, user.getAge());
}
@Override
public int getBatchSize() {
return users.size();
}
});
}
```
In this example, we're inserting a list of `User` objects into a table called `users`. The `jdbcTemplate.batchUpdate()` method takes two arguments: the SQL query string and a `BatchPreparedStatementSetter` object that sets the parameter values for each row in the batch.
The `setValues()` method in the `BatchPreparedStatementSetter` object sets the parameter values for each row in the batch. In this example, we're getting the `User` object at index `i` in the `users` list and setting its `id`, `name`, and `age` properties as the parameter values for the corresponding placeholders in the SQL query string.
The `getBatchSize()` method in the `BatchPreparedStatementSetter` object returns the size of the batch, which is the number of `User` objects in the `users` list.
When the `jdbcTemplate.batchUpdate()` method is called, it executes the SQL query string for each row in the batch with the corresponding parameter values set by the `BatchPreparedStatementSetter` object.
jdbcTemplate.batchUpdate() 中public void add(User user) 的示例
Sure, here's an example of using `jdbcTemplate.batchUpdate()` with a `public void add(User user)` method:
```java
public void add(User user) {
String sql = "INSERT INTO users (id, name, age) VALUES (?, ?, ?)";
jdbcTemplate.update(sql, user.getId(), user.getName(), user.getAge());
}
public void batchInsert(List<User> users) {
String sql = "INSERT INTO users (id, name, age) VALUES (?, ?, ?)";
jdbcTemplate.batchUpdate(sql, new BatchPreparedStatementSetter() {
@Override
public void setValues(PreparedStatement ps, int i) throws SQLException {
User user = users.get(i);
ps.setInt(1, user.getId());
ps.setString(2, user.getName());
ps.setInt(3, user.getAge());
}
@Override
public int getBatchSize() {
return users.size();
}
});
}
```
In this example, we have two methods. The first method, `add(User user)`, inserts a single `User` object into the `users` table using the `jdbcTemplate.update()` method. The SQL query string is the same as in the previous example, and the parameter values are set using the `getId()`, `getName()`, and `getAge()` methods of the `User` object.
The second method, `batchInsert(List<User> users)`, inserts a batch of `User` objects into the `users` table using the `jdbcTemplate.batchUpdate()` method. This method is similar to the previous example, but instead of calling `jdbcTemplate.update()` for each `User` object, we're using a `BatchPreparedStatementSetter` object to set the parameter values for each row in the batch.
Both methods use the same SQL query string, so we don't need to repeat it. We can simply define it once at the beginning of the class.
阅读全文
相关推荐
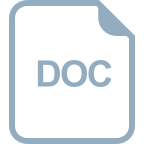
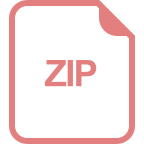
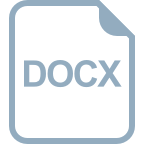
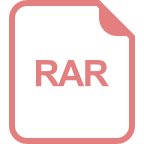
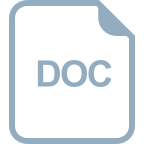








