编写根据十个学生的三科考试成绩算出各学科的总分,平均分数,找出各学科的第一名的程序
时间: 2024-12-03 13:31:25 浏览: 10
在编写这个程序时,我们可以假设有一个学生类(Student),包含姓名(name)、三科成绩(scores = [math, science, english])。首先,我们需要定义一个函数来计算总分、平均分以及找出每个学科的第一名。以下是用Python的一个简单示例:
```python
class Student:
def __init__(self, name):
self.name = name
self.scores = [0, 0, 0]
# 设置成绩
def set_scores(self, math, science, english):
self.scores[0] = math
self.scores[1] = science
self.scores[2] = english
# 定义计算总分、平均分和第一名的辅助函数
def calculate_totals_average_and_first_place(students):
total_scores = [[0, 0, 0] for _ in range(len(students))]
average_scores = [0, 0, 0]
for i, student in enumerate(students):
total_scores[i][0] += sum(student.scores)
average_scores[0] += student.scores[0]
average_scores[1] += student.scores[1]
average_scores[2] += student.scores[2]
for subject_scores in zip(*total_scores):
max_score = max(subject_scores)
max_index = subject_scores.index(max_score)
print(f"学科{max_index+1}的第一名学生是 {students[max_index].name}, 分数为 {max_score}")
# 创建并设置学生数据
student1 = Student("学生A")
student1.set_scores(85, 90, 78)
# 添加其他九位学生的数据...
# 这里省略了具体的创建和设置,实际操作中需要为每位学生创建实例并设置成绩
# 调用计算函数
calculate_totals_average_and_first_place([student1])
```
在这个程序中,你需要为每个学生添加成绩,并将他们作为列表传递给`calculate_totals_average_and_first_place`函数。注意,这只是一个基本的实现,如果你需要处理更多学生或者更复杂的数据结构,可能需要调整类的设计和计算方法。
阅读全文
相关推荐
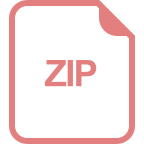
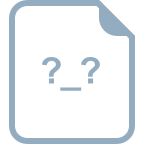
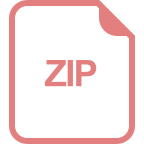















