Android自定义view实现两张图片的分割线滑动对比
时间: 2024-09-24 10:24:37 浏览: 78
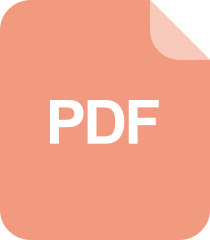
Android 自定义View结合自定义TabLayout实现顶部标签滑动效果
在Android中,如果你想要实现两张图片之间的分割线滑动对比效果,可以创建一个自定义View并结合RecyclerView、DragSortHelper或者其他手势库来完成。以下是一个基本步骤:
1. **创建自定义View**:
创建一个新的布局文件,比如`MySlideView.xml`,在这个视图里,你可以有两个ImageView用于显示图片,并在其之间添加一个`ViewGroup`作为分割线容器。
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:id="@+id/image_view_1"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1" />
<View
android:id="@+id/split_line"
android:layout_width="1dp"
android:layout_height="match_parent"
android:background="@drawable/separator_line" />
<ImageView
android:id="@+id/image_view_2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1" />
</LinearLayout>
```
2. **自定义View Class**:
创建一个自定义的View类(如`MySlideView.java`),覆盖一些必要的方法来处理滑动事件,例如`onTouchEvent`。
```java
public class MySlideView extends LinearLayout {
private ImageView imageView1;
private ImageView imageView2;
private View splitLine;
public MySlideView(Context context) {
super(context);
init();
}
// ...其他构造函数
private void init() {
// 初始化视图组件并设置适配器等
imageView1 = findViewById(R.id.image_view_1);
imageView2 = findViewById(R.id.image_view_2);
splitLine = findViewById(R.id.split_line);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
startDrag(event);
break;
// ...其他触摸事件处理
}
return true;
}
private void startDrag(MotionEvent event) {
DragSortHelper helper = new DragSortHelper(this);
// 实现拖拽逻辑,监听move、dragend事件更新图片位置和分隔线
}
}
```
3. **设置数据和动画**:
将两个ImageView关联到实际的数据源,当滑动操作结束时,可以根据滑动距离调整图片的位置,并可能触发图像对比度的变化或其他视觉反馈。
4. **整合RecyclerView**:
如果你打算在一个RecyclerViev中使用这个自定义View,确保你在ViewHolder中实例化`MySlideView`,并在适配器中处理item的添加、移除和更新。
阅读全文
相关推荐
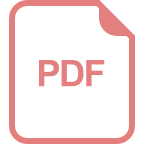
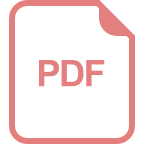
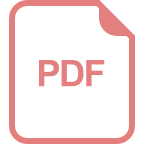
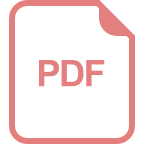
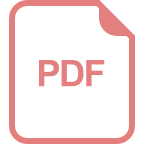
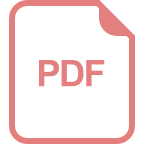
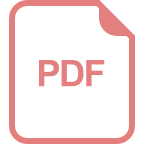
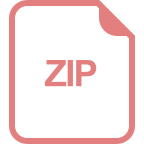
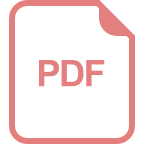
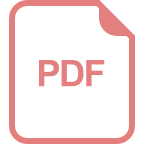
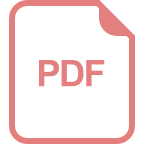
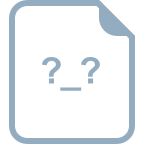
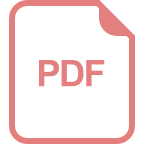
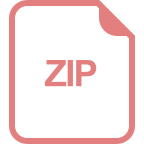