从键盘上输入一个字符串,然后统计其中大写字母、小写字母、数字、空格和其它字符的个数。(用指针实现)
时间: 2024-12-12 09:26:30 浏览: 9
在C语言中,你可以使用指针来实现这个功能。首先,定义一个函数`count_chars(char *str)`,它接受一个指向字符串的指针作为参数。下面是一个简单的步骤:
1. 定义四个整型变量,分别表示大写字母(isUpper)、小写字母(isLower)、数字(isdigit)和非字母数字字符(other)的数量。初始化它们都为0。
```c
int isUpper = 0;
int isLower = 0;
int digit = 0;
int other = 0;
```
2. 创建一个循环,遍历字符串的每一个字符。对于每个字符,可以使用`*str`获取当前字符并检查它的ASCII值:
- 如果是大写字母(ASCII值65到90),加1到`isUpper`。
- 如果是小写字母(ASCII值97到122),加1到`isLower`。
- 如果是数字(ASCII值48到57),加1到`digit`。
- 否则(即不是空格和其他字符),加1到`other`。
```c
for (char *p = str; *p != '\0'; p++) {
if (*p >= 'A' && *p <= 'Z') {
isUpper++;
} else if (*p >= 'a' && *p <= 'z') {
isLower++;
} else if (*p >= '0' && *p <= '9') {
digit++;
} else {
other++;
}
}
```
3. 函数结束时返回这四个计数器的值。
```c
return isUpper + isLower + digit + other;
```
完整代码示例:
```c
#include <ctype.h>
int count_chars(char *str) {
int isUpper = 0;
int isLower = 0;
int digit = 0;
int other = 0;
for (char *p = str; *p != '\0'; p++) {
if (isupper(*p)) {
isUpper++;
} else if (islower(*p)) {
isLower++;
} else if (isdigit(*p)) {
digit++;
} else {
other++;
}
}
return isUpper + isLower + digit + other;
}
// 使用示例
int main() {
char input[] = "Hello, 123 World!";
int result = count_chars(input);
printf("大写字母 %d, 小写字母 %d, 数字 %d, 其他字符 %d\n", result);
return 0;
}
```
阅读全文
相关推荐
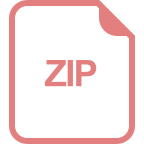
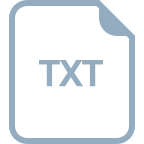
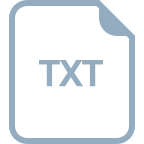















