用D3绘制直方图,该直方图为10个矩形组成,高度设置为随机值,并添加文字图例
时间: 2024-10-21 22:18:35 浏览: 35
在D3.js中,我们可以利用其强大的数据可视化能力创建一个包含10个矩形的直方图,每个矩形的高度基于随机数。以下是简单的步骤:
1. 首先,你需要引入d3库并设置SVG元素作为容器:
```javascript
const width = 600;
const height = 400;
const svg = d3.select('body')
.append('svg')
.attr('width', width)
.attr('height', height);
```
2. 创建随机数组生成10个高度值:
```javascript
const data = Array.from({ length: 10 }, () => Math.floor(Math.random() * 50) + 1); // 高度范围从1到50
```
3. 使用`d3.scaleBand`来设置x轴的分组和宽度:
```javascript
const xScale = d3.scaleBand()
.domain(data.map((_, i) => `bin${i+1}`)) // 假设我们用"bin1", "bin2"等标识每个区间
.range([0, width])
.padding(0.1);
```
4. 创建一个函数来渲染矩形和文本:
```javascript
function renderRects(rectData) {
rectData.forEach((value, index) => {
const y = height - value; // 矩形顶点位置由最大值开始计数
svg.append('rect')
.datum({ [index]: value })
.attr('x', xScale(`bin${index+1}`))
.attr('y', y)
.attr('width', xScale.bandwidth())
.attr('height', value)
.style('fill', 'steelblue'); // 设置颜色
svg.append('text')
.text(`${value}`)
.attr('x', xScale(`bin${index+1}`) + xScale.bandwidth()/2)
.attr('y', y - 5) // 文字下方一点的位置
.attr('dy', '.35em') // 字体对齐
.textAlign('center');
});
}
renderRects(data);
```
5. 调用这个渲染函数,传入你的随机数据。
阅读全文
相关推荐



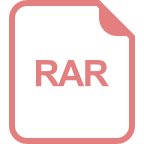














