1)将两个递增的有序链表合并为一个递增的有序链表。要求结果链表仍使用原来两个链表情空间,不另外占用其他的存储空间。表中不允许有重复的数据。用C语言实现
时间: 2024-10-23 15:15:56 浏览: 25
在C语言中,可以采用迭代的方式合并两个递增的有序链表,同时保持原链表节点不被修改。以下是具体的步骤和代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 合并两个递增有序链表函数
Node* mergeSortedLists(Node* list1, Node* list2) {
// 初始化当前指针
Node* current = NULL;
if (list1 == NULL) {
return list2;
}
else if (list2 == NULL) {
return list1;
}
// 判断第一个列表是否小于第二个列表
if (list1->data <= list2->data) {
current = list1;
list1 = list1->next;
} else {
current = list2;
list2 = list2->next;
}
while (list1 != NULL && list2 != NULL) {
// 比较并合并
if (list1->data <= list2->data) {
current->next = list1;
list1 = list1->next;
} else {
current->next = list2;
list2 = list2->next;
}
current = current->next;
}
// 如果其中一个链表已经遍历完,把剩余的那个加到结果链表末尾
if (list1 != NULL) {
current->next = list1;
} else {
current->next = list2;
}
return current;
}
// 测试示例
int main() {
Node* list1 = malloc(sizeof(Node));
Node* list2 = malloc(sizeof(Node));
... // 初始化两个已排序的链表
// 合并链表
list1 = mergeSortedLists(list1, list2);
// 遍历并打印结果
Node* temp = list1;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
free(list1); // 注意释放内存
free(list2);
return 0;
}
阅读全文
相关推荐
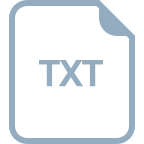
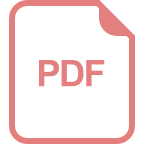
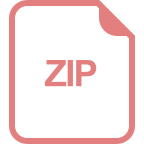















