输入一条英文字符串,先通过程序去除字符串中重复字母并按字典序排列,然后通过递归程序调用形式实现这些字母的所有排列顺序显示。
时间: 2024-12-10 18:51:13 浏览: 5
当你有一个英文字符串,首先需要进行的是去重和排序。这可以通过Python中的set数据结构轻松完成,因为set会自动去除重复元素,然后再转换回列表并排序。例如:
```python
def remove_duplicates_and_sort(s):
s = ''.join(sorted(set(s)))
return s
```
接下来,我们需要生成这个排序后的字符串的所有可能的排列。可以使用itertools模块的permutations函数,它会返回所有可能的排列。这里是一个递归的方式来实现这个功能:
```python
import itertools
def generate_permutations(s):
if len(s) == 0:
return ['']
else:
first_char = s[0]
remaining_chars = s[1:]
sub_perms = generate_permutations(remaining_chars)
return [''.join(p) + first_char for p in sub_perms] + [p + first_char for p in sub_perms]
def main(s):
unique_sorted_s = remove_duplicates_and_sort(s)
permutations = generate_permutations(unique_sorted_s)
print("Sorted and unique string: ", unique_sorted_s)
print("Permutations: ")
for perm in permutations:
print(perm)
# 测试
main("abc")
```
阅读全文
相关推荐
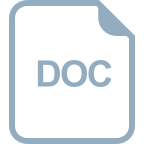
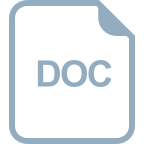
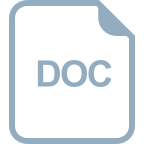
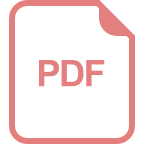




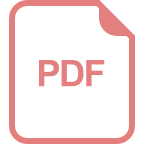
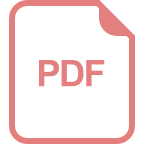
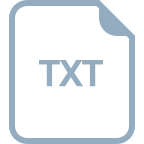
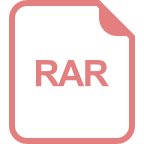
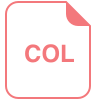
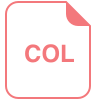



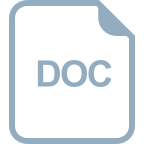
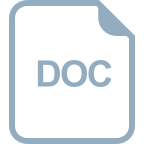