找到单链表中值为Key的元素 将其与前一个结点位置发生互换
时间: 2024-09-22 21:04:36 浏览: 46
在单链表中找到值为`Key`的元素并与其前一个节点交换位置,这个操作通常涉及到遍历链表以及查找目标节点的过程。以下是步骤概述:
1. 首先,创建两个指针,分别命名为`current`和`prev`。`current`初始指向链表的头节点,而`prev`初始化为`None`。
2. 进入循环,当`current`不为`None`时,检查`current`的值是否等于`Key`。如果是,则需要保存当前节点的下一个节点,因为接下来会将它替换掉。
3. 如果找到了目标节点,即`current.value == Key`,那么更新`prev.next`为`current.next`,同时更新`current.next`为之前保存的节点(即`current.next`),实现了两个节点的交换。
4. 更新`prev`为`current`,继续向后遍历直到`current`不再是目标节点。
5. 当遍历结束后,如果未找到匹配值的节点,说明链表中不存在值为`Key`的元素。
以下是Python代码示例(假设链表节点有一个`value`属性和一个`next`引用):
```python
class ListNode:
def __init__(self, value=0, next=None):
self.value = value
self.next = next
def swap_with_previous(head, Key):
current = head
prev = None
while current is not None and current.value != Key:
temp = current
current = current.next
if current is not None:
prev = temp
if current is None or current.value != Key:
return head # 没有找到值为Key的节点,直接返回原链表
# 交换操作
if prev is not None:
prev.next = current.next
current.next = temp
return head
```
阅读全文
相关推荐
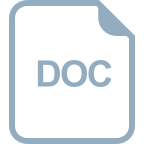
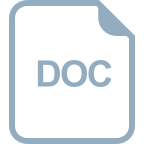
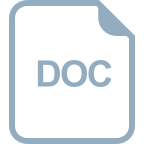





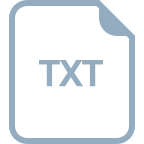
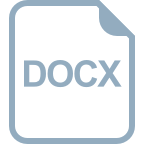
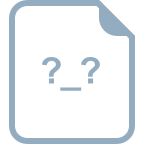
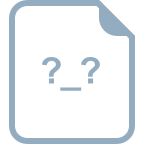
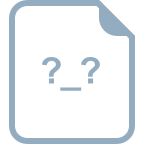




