opencvsharp FindContours
时间: 2024-01-21 10:03:23 浏览: 138
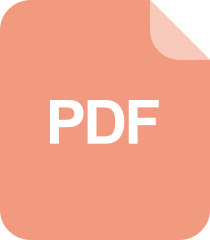
Python通过OpenCV的findContours获取轮廓并切割实例

在C#中使用OpenCvSharp库进行轮廓查找(FindContours)的步骤如下:
1. 首先,确保已经安装了OpenCvSharp库。可以通过NuGet包管理器或者手动下载并添加引用。
2. 导入必要的命名空间:
```csharp
using OpenCvSharp;
```
3. 加载图像:
```csharp
Mat image = Cv2.ImRead("image.jpg", ImreadModes.GrayScale);
```
这里假设要查找轮廓的图像是名为"image.jpg"的灰度图像。
4. 对图像进行预处理(可选):
如果需要对图像进行预处理,例如二值化、平滑等操作,可以在此步骤中进行。这取决于具体的需求。
5. 查找轮廓:
```csharp
Point[][] contours;
HierarchyIndex[] hierarchy;
Cv2.FindContours(image, out contours, out hierarchy, RetrievalModes.External, ContourApproximationModes.ApproxSimple);
```
这里使用了`FindContours`方法来查找轮廓。`RetrievalModes.External`表示只检测外部轮廓,`ContourApproximationModes.ApproxSimple`表示使用简单的逼近方法来表示轮廓。
6. 处理轮廓:
可以根据需要对找到的轮廓进行进一步的处理,例如绘制、计算面积等。
下面是一个完整的示例代码:
```csharp
using OpenCvSharp;
class Program
{
static void Main(string[] args)
{
Mat image = Cv2.ImRead("image.jpg", ImreadModes.GrayScale);
Point[][] contours;
HierarchyIndex[] hierarchy;
Cv2.FindContours(image, out contours, out hierarchy, RetrievalModes.External, ContourApproximationModes.ApproxSimple);
// 处理轮廓
for (int i = 0; i < contours.Length; i++)
{
Cv2.DrawContours(image, contours, i, Scalar.Red, 2);
double area = Cv2.ContourArea(contours[i]);
// 其他处理操作...
}
Cv2.ImShow("Contours", image);
Cv2.WaitKey(0);
}
}
```
请注意,以上代码仅为示例,具体的处理方式和操作根据实际需求进行调整。
阅读全文
相关推荐
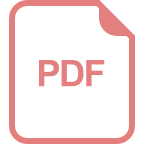





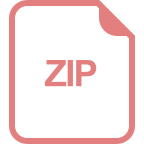








