帮我写一个Python的3D照片旋转编码
时间: 2024-09-13 07:15:06 浏览: 35
在Python中,你可以使用`matplotlib`库来创建一个简单的3D旋转动画。以下是一个示例代码,演示如何创建一个绕y轴旋转的3D立方体的动画。
首先,确保你已经安装了`matplotlib`库,如果没有安装,可以使用pip来安装它:
```bash
pip install matplotlib
```
然后,你可以使用以下Python代码来创建一个3D旋转动画:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
from matplotlib.animation import FuncAnimation
# 创建一个新的图和一个3D子图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 定义一个立方体的8个顶点
vertices = np.array([[1, -1, -1], [1, 1, -1], [-1, 1, -1], [-1, -1, -1],
[1, -1, 1], [1, 1, 1], [-1, 1, 1], [-1, -1, 1]])
# 定义立方体的6个面,每行代表一个面,每列代表一个顶点的索引
faces = np.array([[0, 1, 2, 3], [3, 2, 7, 6], [6, 7, 5, 4],
[4, 5, 1, 0], [1, 5, 7, 2], [4, 0, 3, 6]])
# 绘制立方体的面
def draw_cube(ax, vertices, faces):
for face in faces:
vertices_face = vertices[face]
ax.plot(vertices_face[:, 0], vertices_face[:, 1], vertices_face[:, 2])
# 初始化动画
def init():
ax.set_xlim([-1.5, 1.5])
ax.set_ylim([-1.5, 1.5])
ax.set_zlim([-1.5, 1.5])
ax.set_xticks([])
ax.set_yticks([])
ax.set_zticks([])
return fig,
# 更新动画的函数
def update_cube(frame):
ax.cla() # 清除之前的帧
angle = np.radians(frame) # 将角度转换为弧度
# 应用旋转矩阵绕y轴旋转
rotated_vertices = np.dot(vertices, [[np.cos(angle), 0, np.sin(angle)], [0, 1, 0], [-np.sin(angle), 0, np.cos(angle)]])
draw_cube(ax, rotated_vertices, faces)
return fig,
# 创建动画
ani = FuncAnimation(fig, update_cube, frames=np.arange(0, 360, 1), init_func=init, blit=True)
plt.show()
```
这段代码首先定义了一个立方体的顶点和面,然后通过更新函数`update_cube`对立方体进行旋转,并使用`FuncAnimation`来创建动画效果。
相关推荐
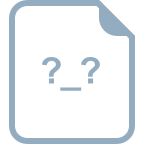
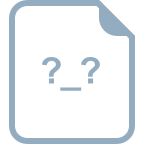
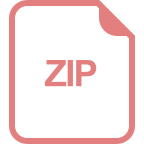














