draw.text(text_position, text, fill='black') 中单纯的调整字体的大小
时间: 2024-12-16 13:28:21 浏览: 41
`draw.text(text_position, text, fill='black')` 是`PIL`库中的一个文本绘制函数,其中`text_position`是文本的开始位置坐标,`text`是要绘制的文字内容,`fill`参数指定字体颜色,默认为黑色。如果你想单纯调整字体大小,你需要在调用这个函数前先创建一个`ImageFont`对象,并传入字体文件名和所需的字体大小,例如:
```python
from PIL import Image, ImageDraw, ImageFont
# 加载特定字体文件
font_path = 'arial.ttf' # 替换为你需要的字体文件路径
font_size = 48 # 调整字体大小为48像素
# 创建Font对象
font = ImageFont.truetype(font_path, font_size)
# 使用ImageDraw对象绘制文本
img = Image.new('RGB', (500, 500), color='white')
d = ImageDraw.Draw(img)
position = (50, 50) # 文本起点位置
d.text(position, '你好世界', fill='black', font=font)
```
在这个例子中,`font_size`变量决定了文字的大小。当你改变这个值,字体就会相应地变大或变小。
相关问题
from tkinter import * import cv2 import numpy as np from PIL import ImageGrab from tensorflow.keras.models import load_model from temp import * model = load_model('mnist.h5') image_folder = "img/" root = Tk() root.resizable(0, 0) root.title("HDR") lastx, lasty = None, None image_number = 0 cv = Canvas(root, width=1200, height=480, bg='white') cv.grid(row=0, column=0, pady=2, sticky=W, columnspan=2) def clear_widget(): global cv cv.delete('all') def draw_lines(event): global lastx, lasty x, y = event.x, event.y cv.create_line((lastx, lasty, x, y), width=8, fill='black', capstyle=ROUND, smooth=True, splinesteps=12) lastx, lasty = x, y def activate_event(event): global lastx, lasty cv.bind('<B1-Motion>', draw_lines) lastx, lasty = event.x, event.y cv.bind('<Button-1>', activate_event) def Recognize_Digit(): global image_number filename = f'img_{image_number}.png' root.update() widget = cv x = root.winfo_rootx() + widget.winfo_rootx() y = root.winfo_rooty() + widget.winfo_rooty() x1 = x + widget.winfo_width() y1 = y + widget.winfo_height() print(x, y, x1, y1) # get image and save ImageGrab.grab().crop((x, y, x1, y1)).save(image_folder + filename) image = cv2.imread(image_folder + filename, cv2.IMREAD_COLOR) gray = cv2.cvtColor(image.copy(), cv2.COLOR_BGR2GRAY) ret, th = cv2.threshold( gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU) # contours = cv2.findContours( # th, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)[0] Position = findContours(th) for m in range(len(Position)): # make a rectangle box around each curve cv2.rectangle(th, (Position[m][0], Position[m][1]), ( Position[m][2], Position[m][3]), (255, 0, 0), 1) # Cropping out the digit from the image corresponding to the current contours in the for loop digit = th[Position[m][1]:Position[m] [3], Position[m][0]:Position[m][2]] # Resizing that digit to (18, 18) resized_digit = cv2.resize(digit, (18, 18)) # Padding the digit with 5 pixels of black color (zeros) in each side to finally produce the image of (28, 28) padded_digit = np.pad(resized_digit, ((5, 5), (5, 5)), "constant", constant_values=0) digit = padded_digit.reshape(1, 28, 28, 1) digit = digit / 255.0 pred = model.predict([digit])[0] final_pred = np.argmax(pred) data = str(final_pred) + ' ' + str(int(max(pred) * 100)) + '%' print(data) font = cv2.FONT_HERSHEY_SIMPLEX fontScale = 0.5 color = (255, 0, 0) thickness = 1 cv2.putText(th, data, (Position[m][0], Position[m][1] - 5), font, fontScale, color, thickness) cv2.imshow('image', th) cv2.waitKey(0) cv2.destroyAllWindows() btn_save = Button(text='Recognize Digit', command=Recognize_Digit) btn_save.grid(row=2, column=0, pady=1, padx=1) button_clear = Button(text='Clear Widget', command=clear_widget) button_clear.grid(row=2, column=1, pady=1, padx=1) root.mainloop()
这段代码是一个Python程序,用于画图并识别手写数字。它调用了许多库,如tkinter、cv2、numpy、PIL和tensorflow。程序创建了一个图形用户界面,包括一个白色的画布和一个按钮。用户在画布上绘制数字后,可以点击按钮来触发识别数字的功能。程序会将绘制的数字保存为图片文件,并加载训练好的模型(mnist.h5)来识别数字。程序最后打印出保存的图片文件的坐标信息。
python下载支持中文的字体
在Python中,你可以通过`pip`安装支持中文的字体库来让程序能够显示或处理中文字符。最常用的是`PIL`(Pillow)库,它内置了一些常用的字体支持。如果你需要特定的中文字体,可以先在网上找到.ttf或.otf格式的字体文件,然后按照下面的步骤操作:
1. 安装Pillow库,如果尚未安装,可以在命令行输入:
```
pip install pillow
```
2. 将下载的字体文件放在你的项目目录下,例如`fonts`目录。
3. 使用Pillow打开字体文件并加载到图像上,比如展示文本:
```python
from PIL import ImageDraw, ImageFont
# 设置字体路径,注意替换为你的实际字体文件路径
font_path = 'fonts/your_font_name.ttf'
# 创建ImageDraw实例,并指定字体
draw = ImageDraw.Draw(Image.new('RGB', (100, 50), "white"))
font = ImageFont.truetype(font_path, size=24)
# 写入中文文本
text = "你好,世界!"
text_width, text_height = draw.textsize(text, font)
position = (10, 20)
draw.text(position, text, fill="black", font=font)
```
运行上述代码后,你应该能看到包含中文的图片。
如果你的应用需要在控制台输出中文,那么可能需要设置终端或IDE的字符集支持,如PyCharm有专门的设置选项来更改编码。
阅读全文
相关推荐
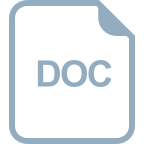
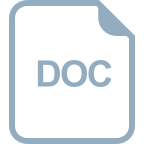
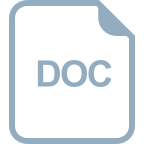
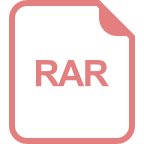
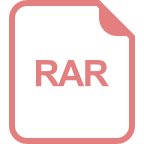
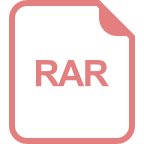
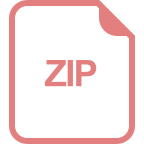
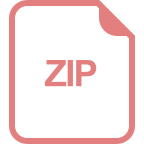
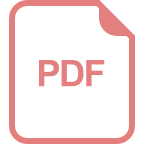
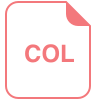
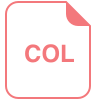
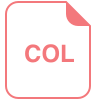
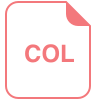
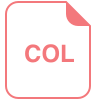
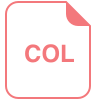

