编写一组程序,基于单链表,用头插法建表,实现某班学生姓名数据的建表、展示、查找,定位,插入,删除、判定表空、求表长等操作用python的解题思路
时间: 2024-09-29 13:14:54 浏览: 35
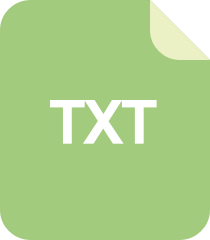
c语言:创建单链表的头插法代码
首先,在Python中,我们可以创建一个简单的单链表结构来存储学生姓名数据。我们将设计一个`Node`类作为节点,包含一个名字属性和一个指向下一个节点的指针,然后创建一个`StudentList`类来管理整个链表。
```python
class Node:
def __init__(self, name=None):
self.name = name
self.next = None
class StudentList:
def __init__(self):
self.head = None
# 插入新学生到链表头部
def insert_at_head(self, name):
new_node = Node(name)
new_node.next = self.head
self.head = new_node
# 展示所有学生
def display(self):
elements = []
current = self.head
while current:
elements.append(current.name)
current = current.next
print("学生列表:", elements)
# 查找学生
def find_student(self, name):
current = self.head
while current and current.name != name:
current = current.next
return current is not None
# 定位学生位置
def position_of_student(self, name):
current = self.head
position = 0
while current and current.name != name:
current = current.next
position += 1
if current:
return position
else:
return -1
# 删除指定学生
def delete_student(self, name):
if self.head is None or self.head.name == name:
self.head = self.head.next
return
prev = None
current = self.head
while current and current.name != name:
prev = current
current = current.next
if current:
prev.next = current.next
# 判定链表是否为空
def is_empty(self):
return self.head is None
# 求链表长度
def length(self):
count = 0
current = self.head
while current:
count += 1
current = current.next
return count
```
阅读全文
相关推荐
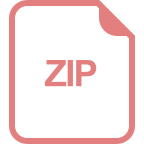
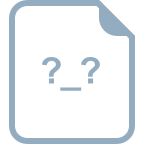





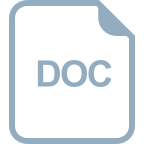
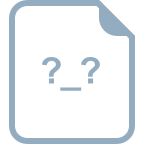
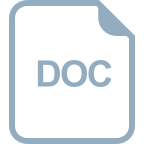
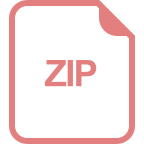
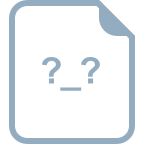
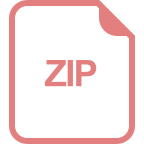
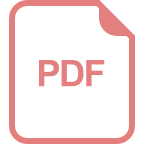
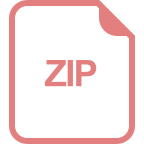
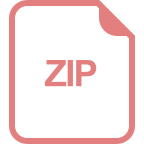