如何在C# .net4.8环境中 Windows Form 应用程序中实现动态每秒中500个数据点更新图形控件(OxyPlot库)的数据? 简单示例代码
时间: 2024-10-22 15:16:30 浏览: 41
在C# .NET 4.8的Windows Forms应用程序中,使用OxyPlot库来实现实时数据更新,你可以通过创建一个`Timer`并在其Tick事件中更新图形控件。以下是一个简单的示例代码:
首先,确保已安装OxyPlot NuGet包。在Visual Studio中打开解决方案管理器,右键点击项目 -> 管理NuGet程序包 -> 搜索"OxyPlot"并安装。
1. 添加必要的命名空间:
```csharp
using OxyPlot;
using OxyPlot.WindowsForms;
using System.Timers;
```
2. 初始化绘图模型和窗口:
```csharp
private PlotModel plotModel;
private Form mainForm;
public MainForm()
{
// 创建一个新的PlotModel实例
plotModel = new PlotModel();
// 创建一个新的WindowsFormsViewHost用于显示OxyPlot图表
var view = new PlotView();
view.Model = plotModel;
// 设置窗体布局
mainForm = new Form { Controls = { view } };
}
```
3. 创建并配置定时器:
```csharp
private readonly Timer timer = new Timer(1000 / 500); // 每500毫秒更新一次
private void InitializeTimer()
{
timer.Elapsed += OnTimerElapsed;
timer.Start();
}
private void OnTimerElapsed(object sender, ElapsedEventArgs e)
{
// 更新数据点并刷新图表
UpdateDataAndRedraw();
}
// 在这里定义一个方法来生成新的随机数据点
private void UpdateDataAndRedraw()
{
// 假设我们有一个列表来存储数据点
List<DataPoint> dataPoints = GenerateRandomDataPoints();
// 更新绘图模型的数据
plotModel.Series.Clear(); // 清除旧数据
var series = new LineSeries { Title = "实时数据" };
foreach (var point in dataPoints)
{
series.Points.Add(point);
}
plotModel.Series.Add(series);
// 刷新图表
view.InvalidatePlot(true);
}
```
4. 定义数据生成函数(这里以随机数为例):
```csharp
private List<DataPoint> GenerateRandomDataPoints()
{
Random random = new Random();
return Enumerable.Range(0, 500).Select(_ => new DataPoint(random.NextDouble(), random.NextDouble())).ToList();
}
```
现在,当启动这个Windows Form应用程序时,它会在每500毫秒内更新一次图形控件,显示新的数据点。记得在`Main`方法中调用`InitializeTimer()`初始化定时器。
阅读全文
相关推荐







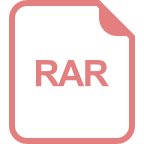


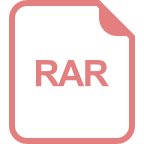






