HttpUtil.post
时间: 2023-09-25 15:15:14 浏览: 178
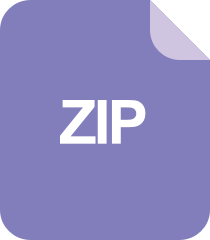
HttpUtil
你可以使用 HttpUtil.post 方法来发送 POST 请求。这个方法通常用于向服务器提交数据或者执行一些操作。你可以提供请求的 URL 和要发送的数据作为参数。具体使用方法如下:
```java
import com.example.util.HttpUtil;
public class Main {
public static void main(String[] args) {
String url = "http://example.com/api";
String postData = "param1=value1¶m2=value2";
String response = HttpUtil.post(url, postData);
System.out.println(response);
}
}
```
在上面的示例中,你需要替换 `url` 变量为你要发送 POST 请求的目标 URL,`postData` 变量为你要发送的数据。`HttpUtil.post` 方法会返回服务器的响应结果,你可以根据需要进行处理或输出。
请注意,上述示例中的 `HttpUtil` 类是一个自定义的工具类,你需要根据实际情况进行替换或编写自己的工具类来实现 POST 请求的发送。
阅读全文
相关推荐














