计算点到多段线的最短距离
时间: 2024-09-11 14:04:19 浏览: 84
计算点到多段线的最短距离可以通过以下步骤实现:
1. 对于每一段线段,使用点到直线段的最短距离公式来计算点到该线段的距离。
2. 确定所有距离中最小的那个,这将是点到多段线的最短距离。
点到直线段的最短距离可以这样计算:
假设直线段的起点为P1(x1, y1),终点为P2(x2, y2),点为P(x, y),那么直线段的向量为P2-P1,点P在直线上的最近点P'的参数t可以由下面的公式得到:
```
t = ((P - P1) · (P2 - P1)) / |P2 - P1|^2
```
其中,`.` 表示向量点积,`|P2 - P1|` 表示向量P2-P1的模。
点P'坐标计算如下:
```
P'(x) = P1(x) + t * (P2(x) - P1(x))
P'(y) = P1(y) + t * (P2(y) - P1(y))
```
如果`t`的值在0到1之间,表示点P'在直线段上,此时点P到线段P1P2的距离是向量PP'的长度:
```
distance = |P - P'|
```
如果`t`小于0,点P'在线段P1P2起点延长线上,此时点P到线段P1P2的最短距离为PP1的长度。
如果`t`大于1,点P'在线段P1P2终点延长线上,此时点P到线段P1P2的最短距离为PP2的长度。
最后,在所有线段中找到最小的点到线段的距离即可。
下面提供一个C++函数的示例,用于计算点到多段线的最短距离:
```cpp
#include <iostream>
#include <cmath>
struct Point {
double x, y;
};
double distance(const Point& p1, const Point& p2) {
return std::sqrt((p2.x - p1.x) * (p2.x - p1.x) + (p2.y - p1.y) * (p2.y - p1.y));
}
double pointToLineSegmentDistance(const Point& p, const Point& p1, const Point& p2) {
double t = ((p.x - p1.x) * (p2.x - p1.x) + (p.y - p1.y) * (p2.y - p1.y)) /
(distance(p1, p2) * distance(p1, p2));
if (t >= 0.0 && t <= 1.0) {
// The point is on the line segment
double dist = std::sqrt((p.x - (p1.x + t * (p2.x - p1.x))) *
(p.x - (p1.x + t * (p2.x - p1.x))) +
(p.y - (p1.y + t * (p2.y - p1.y))) *
(p.y - (p1.y + t * (p2.y - p1.y))));
return dist;
} else {
// The point is not on the line segment
return std::min(distance(p, p1), distance(p, p2));
}
}
double pointToPolylineDistance(const Point& point, const std::vector<Point>& polyline) {
double minDistance = std::numeric_limits<double>::max();
for (size_t i = 0; i < polyline.size() - 1; ++i) {
minDistance = std::min(minDistance, pointToLineSegmentDistance(point, polyline[i], polyline[i + 1]));
}
return minDistance;
}
int main() {
Point point = {1.0, 2.0};
std::vector<Point> polyline = {{0.0, 0.0}, {3.0, 4.0}, {5.0, 0.0}};
std::cout << "The shortest distance is: " << pointToPolylineDistance(point, polyline) << std::endl;
return 0;
}
```
这段代码定义了点到多段线的最短距离计算函数,首先计算点到每一段线段的最短距离,然后找到最小值。
阅读全文
相关推荐
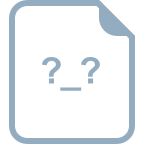
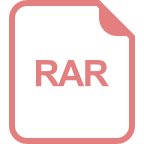
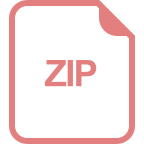
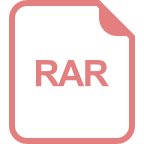
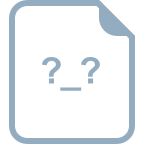
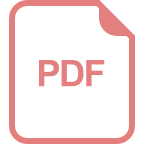
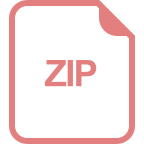
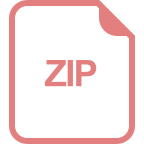
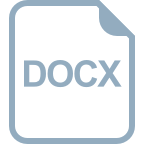
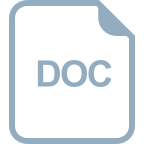
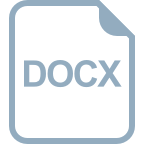
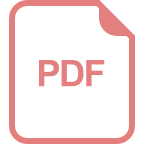
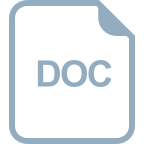



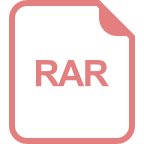