反距离加权插值算法java实现
时间: 2023-09-10 22:11:56 浏览: 68
你可以使用以下代码来实现反距离加权插值算法(Inverse Distance Weighting,IDW)的Java实现:
```java
import java.util.ArrayList;
import java.util.List;
public class IDWAlgorithm {
// 数据点的结构体
private static class DataPoint {
public double x;
public double y;
public double value;
public DataPoint(double x, double y, double value) {
this.x = x;
this.y = y;
this.value = value;
}
}
// 计算两点之间的距离
private static double distance(double x1, double y1, double x2, double y2) {
return Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2));
}
// 反距离加权插值算法
public static double idwInterpolation(List<DataPoint> dataPoints, double x, double y, double power) {
double weightedSum = 0.0;
double weightSum = 0.0;
for (DataPoint point : dataPoints) {
double dist = distance(point.x, point.y, x, y);
if (dist == 0.0) { // 如果点重合,则直接返回该点的值
return point.value;
}
double weight = 1.0 / Math.pow(dist, power);
weightedSum += weight * point.value;
weightSum += weight;
}
if (weightSum == 0.0) {
return 0.0; // 避免除以零错误
}
return weightedSum / weightSum;
}
public static void main(String[] args) {
// 示例数据
List<DataPoint> dataPoints = new ArrayList<>();
dataPoints.add(new DataPoint(0.0, 0.0, 5.0));
dataPoints.add(new DataPoint(1.0, 0.0, 3.0));
dataPoints.add(new DataPoint(0.0, 1.0, 2.0));
// 要插值的坐标
double x = 0.5;
double y = 0.5;
// 插值权重的幂
double power = 2.0;
// 执行插值
double interpolatedValue = idwInterpolation(dataPoints, x, y, power);
System.out.println("插值结果: " + interpolatedValue);
}
}
```
以上是一个简单的反距离加权插值算法的实现示例。你可以根据自己的需求进行调整和扩展。注意,这只是一个基本的实现,可能需要根据实际情况进行改进。
相关推荐
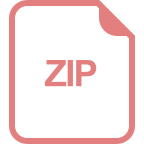














