如何在顺序存储结构和链接存储结构中分别实现一个简单的线性表,并对比它们的时间复杂度差异?
时间: 2024-11-01 22:21:40 浏览: 12
在数据结构的学习中,理解不同存储结构对于线性表的实现及其性能的影响是非常重要的。顺序存储结构通常使用数组实现线性表,而链接存储结构则通常使用链表实现。以下是两种实现方式的详细说明和它们时间复杂度的对比。
参考资源链接:[清华大学王红梅《数据结构》第二版课后答案解析](https://wenku.csdn.net/doc/12tiujg6ti?spm=1055.2569.3001.10343)
顺序存储结构中,线性表的实现依赖于数组。在数组中,数据元素连续存放,逻辑顺序和物理顺序一致。添加和删除操作通常涉及到移动数组中的元素,其时间复杂度为O(n)。然而,访问任一位置的元素的时间复杂度为O(1),因为可以直接通过下标访问。
示例代码(顺序存储结构):
```python
class SequentialList:
def __init__(self):
self.data = []
def insert(self, index, value):
self.data.insert(index, value)
def delete(self, index):
self.data.pop(index)
```
在链接存储结构中,线性表通过链表来实现,链表中的每个节点包含数据域和指针域,指针指向下一个节点。链表的添加和删除操作时间复杂度为O(1),前提是指针指向了具体的操作位置。但是,访问任意位置的元素,需要从头节点开始遍历,其时间复杂度为O(n)。
示例代码(链接存储结构):
```python
class ListNode:
def __init__(self, value=0, next=None):
self.value = value
self.next = next
class LinkedList:
def __init__(self):
self.head = None
def insert(self, index, value):
new_node = ListNode(value)
if index == 0:
new_node.next = self.head
self.head = new_node
return
current = self.head
current_index = 0
while current.next and current_index < index - 1:
current = current.next
current_index += 1
new_node.next = current.next
current.next = new_node
def delete(self, index):
if self.head is None:
return
current = self.head
if index == 0:
self.head = current.next
current.next = None
return
while current.next and index > 1:
current = current.next
index -= 1
current.next = current.next.next
```
总的来说,顺序存储结构更适合频繁的查找操作,而链接存储结构更适合频繁的插入和删除操作。理解这些基础概念和操作对于掌握更复杂的数据结构至关重要。为了更深入地理解这些概念,建议参考《清华大学王红梅 数据结构第二版课后答案解析》,它提供了这些知识点的详细解释和练习题答案,帮助巩固学习成果。
参考资源链接:[清华大学王红梅《数据结构》第二版课后答案解析](https://wenku.csdn.net/doc/12tiujg6ti?spm=1055.2569.3001.10343)
阅读全文
相关推荐
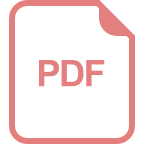
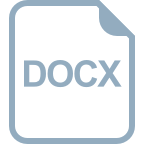
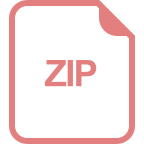
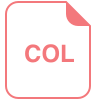









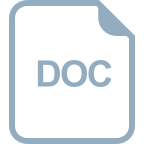




