一维张量 softmax
时间: 2023-12-01 22:42:13 浏览: 95
根据提供的引用内容,可以得知softmax函数是将一个向量转化为概率分布的函数。对于一维张量,可以使用以下代码实现softmax函数:
```python
import torch
def softmax(x):
x_exp = torch.exp(x)
partition = x_exp.sum()
return x_exp / partition
```
其中,x为一个一维张量。使用torch.exp()函数对x中的每个元素进行指数运算,然后对所有指数求和,得到分母partition。最后将每个指数除以partition,即可得到softmax函数的输出,也就是一个概率分布。
以下是一个使用上述softmax函数的例子:
```python
x = torch.tensor([1.0, 2.0, 3.0])
x_prob = softmax(x)
print(x_prob)
```
输出结果为:
```
tensor([0.0900, 0.2447, 0.6652])
```
相关问题
使用tensorflow实现多层神经网络分类鸢尾花任务: 根据右图设计的多层神经网络实现鸢尾花分类任务 提示: 1、隐含层神经元使用relu激活函数 2、输出层神经元使用softmax激活函数 3、W1为输入层与隐含层之间的权重参数,维度是一个二维的 张量(4,16);B1为输入层与隐含层之间的阈值参数,其维 度是一个一维的张量(16,) 。 4、W2为输入层与隐含层之间的权重参数,其维度是一个二维 的张量(16,3);B2为输入层与隐含层之间的阈值参数其维 度是一个一维的张量(3,) 。
好的,这是一个使用tensorflow实现多层神经网络分类鸢尾花任务的示例代码:
```python
import tensorflow as tf
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import OneHotEncoder
import numpy as np
# 加载鸢尾花数据集
iris = load_iris()
X = iris.data
y = iris.target.reshape(-1, 1)
# One-hot编码
encoder = OneHotEncoder(sparse=False)
y = encoder.fit_transform(y)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# 定义模型
model = tf.keras.Sequential([
tf.keras.layers.Dense(16, activation='relu', input_shape=(4,)),
tf.keras.layers.Dense(3, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(X_train, y_train, epochs=50, batch_size=16, verbose=0)
# 评估模型
loss, accuracy = model.evaluate(X_test, y_test, verbose=0)
print('Test accuracy:', accuracy)
```
其中,模型的定义采用了Sequential模型,分别使用Dense层来构建隐含层和输出层。在训练过程中,我们使用adam优化器和交叉熵损失函数来进行模型的优化,同时也输出了模型在测试集上的准确率。
学习使用torch中的Softmax层: 随机生成一个20维的张量,输出其通过Softmax层后的结果
ChitGPT: 可以的,Softmax层是用来将输入数据转换为概率分布的,可以使用torch.nn.Softmax()来实现。以下是代码示例:
```python
import torch
# 随机生成一个20维的张量
x = torch.randn(20)
# 定义Softmax层
softmax = torch.nn.Softmax(dim=0)
# 将x通过Softmax层
output = softmax(x)
print(output)
```
输出结果是一个20维的张量,每一个元素都是概率值,且所有元素的和为1。
阅读全文
相关推荐
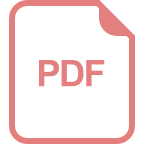
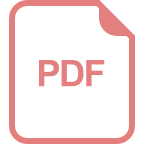
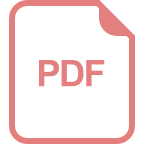
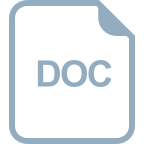












