用C语言的链表写出代码回答文档中的第三和第四个问题
时间: 2024-10-19 13:11:13 浏览: 22
您提到的文档中的实验题目并未明确提及第三个和第四个问题。不过,我假设您是指基于链表实现图书信息表的创建和输出功能。以下是使用链表实现该功能的 C 语言代码:
### 链表节点定义
首先,我们需要定义链表节点结构体 `BookNode` 和相关操作函数。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义图书信息结构体
typedef struct {
char no[20]; // 图书 ISBN
char name[50]; // 图书名字
float price; // 图书价格
} Book;
// 定义链表节点结构体
typedef struct Node {
Book data;
struct Node *next;
} BookNode;
// 创建新节点
BookNode* createNode(const char *no, const char *name, float price) {
BookNode *newNode = (BookNode *)malloc(sizeof(BookNode));
if (newNode == NULL) {
printf("内存分配失败\n");
exit(1);
}
strcpy(newNode->data.no, no);
strcpy(newNode->data.name, name);
newNode->data.price = price;
newNode->next = NULL;
return newNode;
}
// 插入节点到链表末尾
void insertAtEnd(BookNode **head, BookNode *newNode) {
if (*head == NULL) {
*head = newNode;
} else {
BookNode *current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 统计链表中的节点数量
int countNodes(BookNode *head) {
int count = 0;
BookNode *current = head;
while (current != NULL) {
count++;
current = current->next;
}
return count;
}
// 输出链表中的所有节点
void printList(BookNode *head) {
BookNode *current = head;
while (current != NULL) {
printf("%s %s %.2f\n", current->data.no, current->data.name, current->data.price);
current = current->next;
}
}
// 主函数
int main() {
BookNode *head = NULL;
char no[20], name[50];
float price;
while (1) {
scanf("%s %s %f", no, name, &price);
if (strcmp(no, "0") == 0 && strcmp(name, "0") == 0 && price == 0.0) {
break;
}
BookNode *newNode = createNode(no, name, price);
insertAtEnd(&head, newNode);
}
int count = countNodes(head);
printf("%d\n", count);
printList(head);
// 释放链表内存
BookNode *current = head;
while (current != NULL) {
BookNode *temp = current;
current = current->next;
free(temp);
}
return 0;
}
```
### 代码说明
1. **节点定义**:`BookNode` 结构体包含图书信息和指向下一个节点的指针。
2. **创建节点**:`createNode` 函数用于创建新的链表节点。
3. **插入节点**:`insertAtEnd` 函数将新节点插入到链表的末尾。
4. **统计节点数量**:`countNodes` 函数返回链表中节点的数量。
5. **输出链表**:`printList` 函数逐行输出链表中的每个节点信息。
6. **主函数**:从标准输入读取图书信息,直到遇到输入结束标志 `0 0 0`,然后输出图书总数和每本书的信息,并释放链表占用的内存。
希望这段代码能满足您的需求。如果有任何进一步的问题或需要调整的地方,请随时告知。
阅读全文
相关推荐
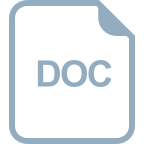
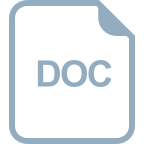
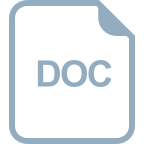
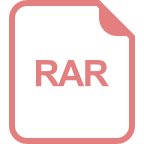

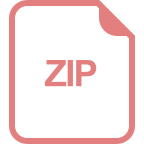
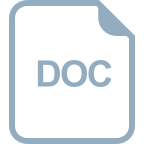
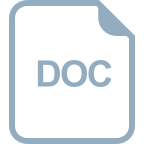
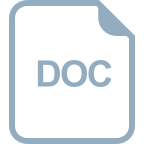
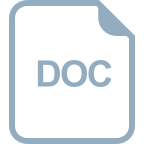
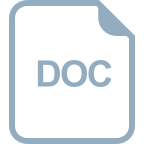
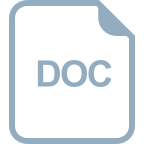
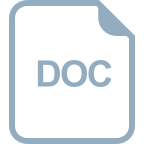
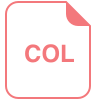
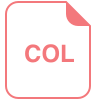
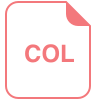
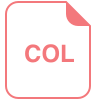
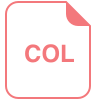
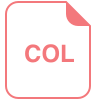