给定一个n*m的字符矩阵,矩阵中每个元素都是大写或小写字母。程序需要统计每个字母出现的次数(不区分大小写),并按出现次数从高到低进行排序,如果字母出现次数相同,则按字典序排序。按顺序输出前5个字母及其出现次数(输出时所有字母统一为小写)c++
时间: 2024-10-28 19:06:57 浏览: 19
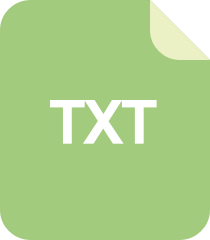
密码学-字母统计

为了实现这个功能,你可以按照以下步骤编写一个 C++ 程序:
1. 定义一个 `std::map<char, int>` 或者 `std::unordered_map<char, int>` 来存储每个字母及其出现的次数。`std::map` 提供了自动排序的功能,而 `std::unordered_map` 则提供更快的查找速度。
```cpp
#include <iostream>
#include <string>
#include <map>
#include <algorithm>
std::pair<std::string, int> sortAndCountLetters(std::vector<std::string>& matrix) {
std::map<char, int> letterCount;
for (const auto& row : matrix) {
for (char c : row) {
char lowerCase = tolower(c); // 转换为小写并累加计数
letterCount[lowerCase]++;
}
}
// 对map中的元素进行排序
std::vector<std::pair<char, int>> sortedCounts(letterCount.begin(), letterCount.end());
std::sort(sortedCounts.begin(), sortedCounts.end(),
[](const auto& a, const auto& b) { return a.second > b.second || (a.second == b.second && a.first < b.first); });
// 取出前五个出现次数最多的字母和对应的计数
if (sortedCounts.size() >= 5) {
return std::make_pair(" ".join(sortedCounts.begin() + 5, " ..."), 5);
} else {
return std::make_pair("".join(sortedCounts.begin(), " "), sortedCounts.size());
}
}
```
2. 主函数中,读取输入矩阵,并调用 `sortAndCountLetters` 函数:
```cpp
int main() {
std::vector<std::string> matrix = {/* 输入的 n*m 矩阵 */};
auto result = sortAndCountLetters(matrix);
std::cout << "Top 5 most common letters (in lowercase): " << result.first << std::endl;
std::cout << "Total count: " << result.second << std::endl;
return 0;
}
```
注意:上述代码假设输入的矩阵已经被初始化并且包含有效的字符。在实际应用中,你需要处理可能出现的边界条件和错误情况。
阅读全文
相关推荐
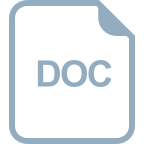
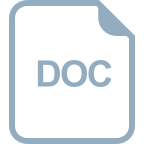
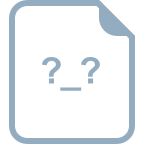
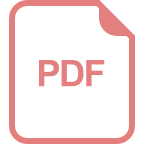
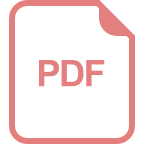
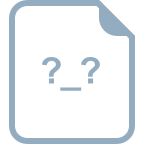
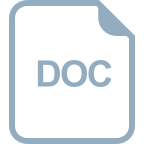
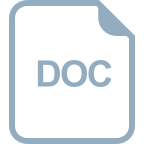
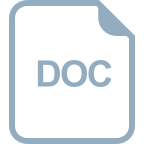
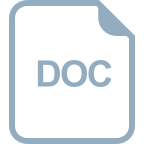
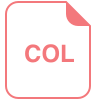
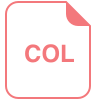
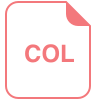
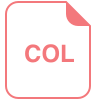
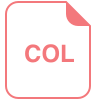
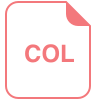

